Socket programming in Java - Table of Content
- What are Socket Programming in Java
- What Is Java Socket
- Client-Side Programming
- Server-Side Programming
- Testing The Applications
- Conclusion
What are Socket Programming in Java
Socket programming is a method where two nodes on a network can connect and communicate with one another. While another socket reaches out to the first socket to establish a connection, one socket (or node) listens on a specific port at an IP address.
While the customer reaches out for the server, the server creates the listener socket. For connection-oriented socket programming, the classes Socket and Server Socket are utilized.
Programming using Java Sockets can be either connection-oriented or connection-less. For connection-oriented socket programming, While the customer reaches out for the server, the server creates the listener socket. For connection-oriented socket programming, the classes Socket and Server Socket are utilized. and DatagramSocket classes are used, while Socket and ServerSocket are used for connection-less socket programming.
What Is Java Socket
A Java socket is one terminal of a 2-way networked communication relationship between two programs. For the TCP layer to recognize the program that data is intended to be transferred to, a socket is tied to a port number.
A port number and an IP address make up an endpoint. An implementation of one side of a 2-way connection between the Java program and another program on the network is made possible by the class Socket, which is part of the Java platform's package. The class resides on top of the platform-specific implementation, shielding your Java program from the specifics of every given system. Your Java programs can interact over the web in a platform-independent manner by utilizing the class rather than depending on native code.
Client-Side Programming
When using the client side, in the programming, the client initially watches for the server to launch. The requests will be sent to the server once it is operational. The client will then watch for the server's answer. So, this is how server and client communication functions overall. Let's now go deeper into client-side and server-side programming.
For starting with the requests from the client-side, the user needs to process the following steps:
Establish a connection :
Creating a socket connection is the initial action. The socket connection signifies that the 2 machines are aware of each other's IP address and TCP port on the network.
The following statement will let you construct a socket:
Socket s = new s(“127.0.0.1”, 5000)
The first input in this case denotes the server's IP address.
The TCP Port is represented by the second parameter. (A number that indicates which server-side program should be running.)
Communication :
Streams are utilized for both data input and output when communicating via a socket connection. You must shut down the connection after opening it and sending the requests.
Closing the connection :
Once the message has been transmitted to the server, the socket connection will explicitly close.
Wish to make a career in the world of Java? Start with HKR'S Java Training !
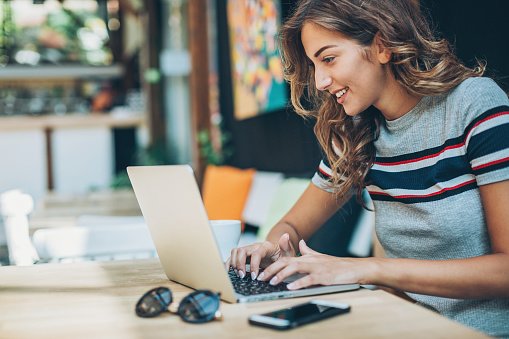
Java Certification Training
- Master Your Craft
- Lifetime LMS & Faculty Access
- 24/7 online expert support
- Real-world & Project Based Learning
Server-Side Programming
In essence, the server will create its object and await a request from the client. The server will reply with the response once the client will send the request.
Two sockets are required in order to program the server-side application, and they are as follows:
When a client calls newSocket(), a ServerSocket that is waiting for the requests from the client is created. There is a straightforward socket for client communication.
Following that, you must inform the client of the outcome.
Communication
The output is sent across the socket using the getOutputStream() function.
Closing the connection
Once everything is finished, it's crucial to shut off the connection by shutting the socket and any active input/output streams.
- You can run the server-side program first after configuring the client and server ends. After that, you must transmit the request and start client-side software. The server will reply as the client sends the request. The image below shows the same.
- The client will establish a connection and enter the request as a string.
- The server will reply to the request sent by the client.
You must run a Java socket program in the given manner. These programs can also be run via a command window or terminal. However, as Eclipse is very feature-rich, you can easily run both apps on a console.
Top 30 frequently asked JAVA Interview Questions !
Subscribe to our YouTube channel to get new updates..!
Testing The Applications
The testing of applications is done using the IntelliJ application or any other IDE.
- Put the two programs together.
- Start the client application after starting the server software.
- Write a message in the client window, and the server window will simultaneously receive and display them.
- Type BYE to leave.
This can be done using a command prompt also:
- Create a new folder called project (this is the name of your package).
- Place the project folder's Server.java and Client.java files.
- Go to the root path on the command prompt after opening it.
- Run java project.Server first, then javac projectServer.java.
- Use the same process to run the client and server programs.
- Messages can then be typed in the window of the client.
The application can fail when a port has already been in use. Modify port no to a special number to resolve this problem.
Acquire Jenkins certification by enrolling in the HKR Jenkins Training program in Hyderabad!
Conclusion
In this article, we have talked about socket and socket programming. Socket programming is a method where two nodes on a network can connect and communicate with one another. While another socket reaches out to the first socket to establish a connection, one socket (or node) listens on a specific port at an IP address. We have also discussed client-side and server-side along with testing the applications.
Related Article:
About Author
As a content writer at HKR trainings, I deliver content on various technologies. I hold my graduation degree in Information technology. I am passionate about helping people understand technology-related content through my easily digestible content. My writings include Data Science, Machine Learning, Artificial Intelligence, Python, Salesforce, Servicenow and etc.
Upcoming Java Certification Training Online classes
Batch starts on 10th Jul 2025 |
|
||
Batch starts on 14th Jul 2025 |
|
||
Batch starts on 18th Jul 2025 |
|
FAQ's
A Java socket is one terminal of a 2-way networked communication relationship between two programs. For the TCP layer to recognize the program that data is intended to be transferred to, a socket is tied to a port number.
The two types of sockets are connectionless and connection-oriented.
Learning socket programming is worthwhile if the learner wants to pursue a career in networking. One out of every thousand computer professionals attempts to know something about it, despite the fact that it is generally seen as a less important field.
There are three types of sockets created in Java which are raw sockets, datagram sockets, and stream sockets.
Yes, socket programming is a point-to-point connection.