List to String in Python - Table of Content
- Creating Lists in Python
- String in Python
- List to string in Python
- Conversion Need from list to string
- Deleting Lists in Python
- List Items
- Conclusion
The main use of lists in python is their capacity to store multiple values in a single variable or we can also say multiple data items in a single data type. They are always present in an ordered fashion containing arbitrary objects. Lists have always been known for their dynamic features as well as mutable nature. In this article, we will understand what lists in python are, how we can create lists in python, how we can change elements in a list, how to delete a formed list, and the list to string method in python.
Become a Python Certified professional by learning this HKR Python Training!
Creating Lists in Python
Lists in python are created using square brackets ([ ]) and the list items are separated using commas.
Below is a python program to create a simple list and get the output.
list1 = ["Welcome", "To", "HKR"]
print(list1)
Output:
['Welcome', 'To', 'HKR']
It is not necessary that a list can have only one data type. Lists can have as many items as the user wants along with different data types such as string, int, float, etc. Check out the python code below where the list is composed of more than one data type.
list1 = []
list1 = [10, "HKR", 11.2]
print(list1)
Output:
[10, "HKR", 11.2]
String in Python
Strings are the data types which is a sequence of characters present inside double-quotes. In python, we have built-in functions to perform operations on strings. Such as if a user wants to concatenate two strings, it can perform it by using the ‘+’ sign. For example, perform concatenation of two words: ‘welcome’ + ‘to HKR’. The output will be welcome to HKR.
Below is a python code to understand strings in python:
str = "Welcome to HKR training"
print(str)
x = '''How may we help you?
string'''
print(x)
Output:
Welcome to HKR training
How may we help you?
string
>
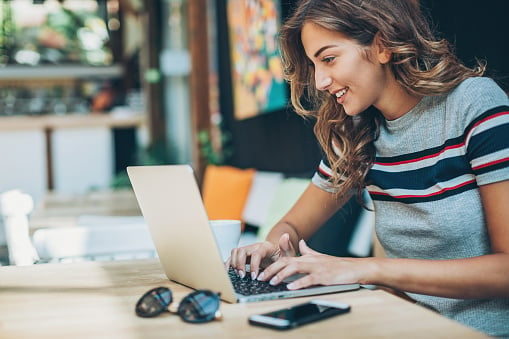
Python Training Certification
- Master Your Craft
- Lifetime LMS & Faculty Access
- 24/7 online expert support
- Real-world & Project Based Learning
List to string in Python
Python lists are the data types that help a user work on multiple items at a single time. However, in day-to-day life, a programmer may face a situation while programming where he needs to code something to the text file, command line, or CSV file. The most appropriate way to conduct this is by using Strings. However, a number of programmers prefer using lists in their programs to store values or variables. So, when there comes a situation where list items need to be converted to a text file, the user needs to first convert the python lists to strings.
There are mainly 4 ways to convert a list to a string in python. Let us have a look at them below:
1. Using Join Function
In python, the join function is one of the easiest methods for converting a list to a string in python. The main thing to acknowledge while executing this function is that the lists should contain only strings as their elements for converting lists into a string using the join function.
Let us see the example below and see how we can convert list to string in python using the join function.
list1 =["Welcome","To","HKR"]
print(",".join(list1))
list1 =["Welcome","To","HKR"]
print("\n".join(list1))
Output:
Welcome,To,HKR
Welcome
To
HKR
This join function method will fail to work in case the list will consist of non-string items such as integers.
2. Traversal of a function
In this, the first step is to declare the list that is to be converted to the string. Then initial the string which will store the elements. Every item in the list will be traversed using a for loop and as it finds an index, the element will be added to the string. In the end using print() function, the list will be printed.
Let us see the example below and see how we can convert a list to a string in python using the transverse method.
list = ['Welcome','To','HKR']
mystring= ' '
for x in list:
mystring += ' ' + x
print(mystring)
Output:
Welcome To HKR
3. Using map() Function
The map function in python can be used on lists if it has two cases:
If the list has different kinds of elements, meaning the list is heterogenous.
If the list consists of numbers only.
Here we use str() function which converts the given data type into string.
Let us see an example below to convert a list to string using map() function.
list1 = [100,200,300,400]
print(str(list1).strip('[]'))
Output:
100, 200, 300, 400
Below is another example below for the second case of map() function to convert list to string in python.
list = ['Welcome','To','HKR']
mystring= ' '.join(map(str, list))
print(mystring)
Output:
Welcome To HKR
4. List Comprehension
This is a method for converting list to string in python where python creates a list of elements from a pre-existing list. It will take in the ‘for’ loop which will traverse the iterable objects in a pattern working element-wise. The best combination for this process is using list comprehension along with jin() function. Each element of the list will be traversed individually and then join() function will concatenate the elements together.
Below is another example below for the second case of map() function to convert a list to string in python.
x = ['Welcome', 'To','HKR']
listToStr = ' '.join([str(elem) for elem in x])
print(listToStr)
Output:
Welcome To HKR
Subscribe to our YouTube channel to get new updates..!
Conversion Need from list to string
The main objective for converting list items to strings is when the user needs to display the list contents in the form of a string. The other most common reason for doing this is string manipulation. It is a process of analysing or handling strings in python. There are various processes involved in string manipulation such as interpreting strings, parsing strings, concatenating two strings, removing string characters from both ends, etc.
Become a IRON Python Certified professional by learning this HKR Ironpython Training!
Deleting Lists in Python
There are various ways to delete the lists in python. The first method is using the clear() function.
Below is a python program to create a simple list and get the output.
list1 = [10, 20, 30, 40]
print('List before cleaning:', list1)
list1.clear()
print('List after clearing:', list1)
Output:
List before cleaning: [10, 20, 30, 40]
List after clearing: []
>
Another method to delete a list is by re-initializing the scope of the list. This is done by initialising the size of the list to 0.
Let is understand this better using the example below:
list1 = [10, 20, 30]
list2 = [50, 60, 70]
print ("List1 before deleting is : "
+ str(list1))
list1.clear()
print ("List1 after deleting using clear() : "
+ str(list1))
print ("List2 before deleting is : "
+ str(list2))
list2 = []
print ("List2 after deleting using reinitialization : "
+ str(list2))
Output:
List1 before deleting is : [10, 20, 30]
List1 after deleting using clear() : []
List2 before deleting is : [50, 60, 70]
List2 after deleting using reinitialization : []
>
The third and least known method for deleting a list is using “*= 0”. This method tends to remove all elements from the list and makes it totally empty.
Below is a python code to execute this method. Have a look:
list1 = [10, 20, 30]
print ("List before deleting will be : " + str(list1))
list1 *= 0
print ("List1 after deleting using this method is: " + str(list1))
Output:
List before deleting will be : [10, 20, 30]
List1 after deleting using this method is: []
>
The last and forth method for deleting a list is using the del function. This method tends to delete a range of elements in a list. If no range is specified, this method will delete all the elements of the list.
Below is a python code to execute the del method in python. Have a look:
list1 = [10, 20, 30]
list2 = [40, 50, 60, 70]
print ("List1 before deleting the elements will be: " + str(list1))
del list1[:]
print ("List1 after deleting using del : " + str(list1))
print ("List2 before deleting will be : " + str(list2))
del list2[:]
print ("List2 after deleting using del : " + str(list2))
Output:
List1 before deleting the elements will be: [10, 20, 30]
List1 after deleting using del : []
List2 before deleting will be : [40, 50, 60, 70]
List2 after deleting using del : []
List Items
There are 3 types of list items under the list category. They are ordered, changeable and list which allows duplicates. If the user wishes to add a new item to the list, it will be added at the end of the list and not in the front. Only a few list methods can change the order of the items in the list, otherwise, it is not possible in general.
Let us see an example below where a list item is made duplicate:
list1 = ["Welcome", "To", "HKR", "To", "HKR"]
print(list1)
Output:
['Welcome', 'To', 'HKR', 'To', 'HKR']
Conclusion
In this article, we have thoroughly described the concepts of lists such as what are lists, how to create lists in python and how the user can change list to string along with the needs to perform this method. We have discussed what are lists in python sling with its types and how to declare them and then delete them. Then we discussed strings and how strings are different from lists. Moving towards the end, we also discussed the 4 main methods with the help of which user can change a list to a string. Those methods are using join() function, list comprehension, traversal of a list and by using map() function.
Related Articles:2. Python Ogre
About Author
As a content writer at HKR trainings, I deliver content on various technologies. I hold my graduation degree in Information technology. I am passionate about helping people understand technology-related content through my easily digestible content. My writings include Data Science, Machine Learning, Artificial Intelligence, Python, Salesforce, Servicenow and etc.
Upcoming Python Training Certification Online classes
Batch starts on 21st Nov 2024 |
|
||
Batch starts on 25th Nov 2024 |
|
||
Batch starts on 29th Nov 2024 |
|