Python Variables - Table of Content
- Python Variable
- Identifier Naming
- Declaring a Variable in Python
- Object Identification Using Variables
- Variable Names
- Python Variable Types
- Variable type in Python
- Object Reference
- Multiple Assignment
- Deleting a Variable
- Conclusion
Python Variable
A name that is used to refer to the memory location in a programming language is called a variable. Python Variables are also termed storage containers in other words. Variables in python are ‘statically typed’ meaning a user does not need to create variables while coding. The variables get declared themselves whenever a value is assigned to them. There are 4 main types of variables: integer, long integer, string, and float. Hence, we cannot have any type of command which can create a variable.
The main use of variables in python is to store values as a reserved memory container. In this article, we will understand what variables actually are, how identifier naming is done while working with the variables, declaration of a variable in python, identifying objects using variables, different types of variable names and their types such as local variable, global variable, object reference and how to finally delete a variable once created.
Become a Python Certified professional by learning this HKR Python Training!
Identifier Naming
Variables in python are just an example of an identifier that will recognize the literals which are being used in the program. They work according to a set of rules which are mentioned below:
- The name of an identifier is always case-sensitive. For example, ‘WelcomeToHKR’ and ‘WelcometoHKR’ are not the same.
- The identifier’s initial character should either be an alphabet or an underscore(_)
- The alphabet following the initial alphabet
- The identifiers cannot have special characters in them including white spaces.
- The name of the identifier need not be the same as the keyword defined in the programming language.
- Some examples to correct identifiers are: x301, _x, x_0, etc.
- Some examples of incorrect identifiers are 2y, 1%r, =34, etc.
Declaring a Variable in Python
As we have discussed, there is no need to create a variable unless there needs to be a value assigned to it. One more thing which is very important to note is that variables need not be declared in a specific type. The type of variable can even be changed after the user declares them. We use the equals (=) operator to assign a value to the variable.
Let us take an example of python code below to understand how we can declare variables in python:
a = 10
b = "HKR"
print(a)
print(b)
Output:
10
HKR
The user can also re-declare the variable after creating it. Check out the python code below:
Number = 10
print("Before declaring the variable: ", Number)
Number = 12
print("After re-declaring the variable:", Number)
Output:
Before declaring the variable: 10
After re-declaring the variable: 12
Object Identification Using Variables
Every variable created in python is unique. It is not possible to have two same variables for 2 different objects. There is a built-in function in python id() which identifies the id of the variable meaning whether it’s defined already or it’s new.
Let us take an example of python code below and understand how object identification is done in Python using variables:
x = 10
y = x
print(id(x))
print(id(y))
x = 50
print(id(x))
Output:
9756512
9756512
9757792
Here in the code above, the user has assigned y = x, where both x and y are pointing to the same object. With the use of id() function, it will also return the same number.
Hence, we will re-assign x to 50; then it is termed as a new object identifier and will have a new changed output.
Variable Names
We have already discussed how variables work with programming languages and how we can declare them along with assigning value to them. The names of variables may be of any length having a lowercase (a to z), an uppercase (A to Z), any digits from 0 to 9, or an underscore (_).
Let us take an example below and see how variable names can work in python.
Name = "Y"
name = "X"
naMe = "Z"
NAME = "M"
n_a_m_e = "L"
_name = "N"
n_a_m_e = "L"
name_ = "O"
_name_ = "P"
na56me = "R"
print(Name,name,naMe,NAME,n_a_m_e, NAME, _name, n_a_m_e, name_,_name, na56me)
Output:
Y X Z M L M N L O N R
As we can see in the example above, the user has declared some valid variable names such as naMe, _name, etc. But this procedure might create confusion when one reads the code so therefore this is not mostly recommended. The user should try making the variable name a little descriptive hence making the code more readable.
The multi-keywords can be created as:
Pascal Case - In this, the first word is capitalized along with the word or abbreviation in the middle of the word. For example: WelcomeToHKR, HowAreYou, etc.
Snake Case - The words are separated using underscore(_) in the snake case. For example Welcome_To_HKR, How_Are_You, etc.
Camel Case - Mostly like the pascal case, each word in the middle will begin with a capital letter. For example: welcomeToHKR, howAreYou, etc.
Acquire Apache NIFI certification by enrolling in the HKR Apache NIFI Training program in Hyderabad!
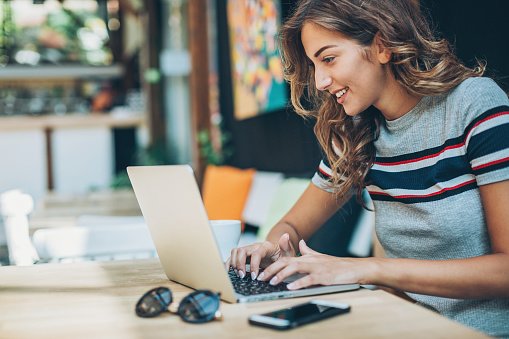
Python Training Certification
- Master Your Craft
- Lifetime LMS & Faculty Access
- 24/7 online expert support
- Real-world & Project Based Learning
Python Variable Types
There are two types of variables in python: Local variable and Global variable.
Let us understand more about these and understand them in depth.
1. Local Variables: These types of variables are always defined inside the function. Their scope is also limited to the function only. Check out the example below of how we can make use of local variables in a python code:
def add():
x = 10
y = 20
z = x + y
print("The sum of numbers is:", z)
add()
Output:
The sum of numbers is: 30
As we can clearly see in the code above, the user declared a function as add() to assign it to variables within the function. The variables will be called local variables as they will have scope inside the function only. If the user tries to declare them outside of the function, he will get a code error called NameError: name 'x' is not defined
2. Global Variables: These types of variables can be used both inside as well as outside the function. Their scope lies in the complete program. In case it is not mentioned in the code, the global variables are by default declared outside the function. In case the user forgets to mention the variable type, it will be local by default. Check out the example below of how we can make use of global variables in a python code:
a = 10
def mainFunction():
global a
print(a)
a = 'Welcome To HKR Training'
print(a)
mainFunction()
print(a)
Output:
10
Welcome To HKR Training
Welcome To HKR Training
As we can see in the code above, the user has declared a global variable a and a value is assigned to it. Then the user defines a function and it accesses the pre-declared variable inside the function by making use of the global keyword.
Variable type in Python
The data types in a programming language basically states the operations that are to be performed on the given data. As we know that python works for objects in the programming, variables work as the objects only for the data types.
Here is a list of few data types that work with python variables:
- Numeric
- Sequence
- Boolean
- Set
- Dictionary
assigned to different values.
Let us consider an example below and see how we can use various data types with variables:
var1 = 12345
print("Numeric data is : ", var1)
String = 'Welcome to HKR'
print("Topic is Python Variables")
print(String)
print(type(True))
print(type(False))
set = set("HKR Trainings")
print("\nSet with the use of String: ")
print(set)
Dict1 = {1: 'HKR', 2: 'Welcomes', 3: 'You'}
print("\nDictionary with the use of Integer Keys: ")
print(Dict1)
Output:
Numeric data is : 12345
Topic is Python Variables
Welcome to HKR
<class 'bool'>
<class 'bool'>
Set with the use of String:
{'g', 'a', 'r', 'R', 'i', 'n', 'T', ' ', 's', 'X', 'H'}
Dictionary with the use of Integer Keys:
{1: 'HKR', 2: 'Welcomes', 3: 'You'}
Top 30 frequently asked Python Interview Questions!
Subscribe to our YouTube channel to get new updates..!
Object Reference
a=10
b=a
If we take the example above, we understand that the code creates an object to represent the value 10. Then, it is creating the variable in case it does not exist. It is made as a reference to this new object having a value of 10. In the second line, there is a creation of another variable b however it isn’t assigned with a but is made in reference to that object that an actually does.
Multiple Assignment
In python, a user is allowed to assign a single value to multiple variables. The user can perform multiple assignments in two different ways. It is done either by assigning one value to different variables or can also be done by having different variables assigned to different values.
Let us see an example below of how we can assign single value to multiple variables:
a=b=c=10
print(a)
print(b)
print(c)
Output:
10
10
10
Now let us see another example of how we can assign multiple values to multiple variables:
a, b, c = 10, 20, "WelcomeToHKR"
print(a)
print(b)
print(c)
Output:
10
20
WelcomeToHKR
As the variables appear, the values will be assigned in the same manner only.
Deleting a Variable
A variable can be deleted using the ‘del’ keyword.
Let us see an example of how we can delete a variable using python:
a = 10
print(a)
del a
print(a)
Output:
Traceback (most recent call last):
File "./prog.py", line 4, in
NameError: name 'a' is not defined
Conclusion
Through this article, we have understood what a variable is, how we can declare a variable inside a function and how we can assign a value to the variable. The article will help you clear all your doubts about python variables along with the basic rules that variables come up with.
Related Articles
About Author
As a content writer at HKR trainings, I deliver content on various technologies. I hold my graduation degree in Information technology. I am passionate about helping people understand technology-related content through my easily digestible content. My writings include Data Science, Machine Learning, Artificial Intelligence, Python, Salesforce, Servicenow and etc.
Upcoming Python Training Certification Online classes
Batch starts on 22nd Jun 2025 |
|
||
Batch starts on 26th Jun 2025 |
|
||
Batch starts on 30th Jun 2025 |
|