Python Pop - Table of Content
- Introduction to pop() in python
- How does a pop() function work?
- Python List pop() Example
- Python Dictionary pop()
- Python Set pop()
- Conclusion
- FAQ’s
Introduction to pop() in python
pop() is a function in computer languages used by programmers to pop an item out of the python list. It actually belongs to a list data type. As we are aware, that list is one of the most widely used python data types. The pop method also aims to remove an item out of an index of the list and return the item that is removed. Therefore, pop() will actually be a list without the popped element.
Become a Python Certified professional by learning this HKR Python Training!
Syntax of pop() in python
Below is the syntax of how a user can pop out an element from the list using pop()in python:
NameOfList.pop(index)
The index above represents a particular element that has to be popped out from the list. When an element is present in the argument of the list from where the element has to be popped out, in case there is no index specified or defined, the last element of the list will be removed using the pop() function. So the user can simply remove the last element of the list comprehend as no argument will be passed on as -1 and will be popped out.
On the other hand, if the user specifies an index that is not in range, then it will throw an IndexError exception.
How does a pop() function work?
- The pop() method cannot accept more than one argument for the execution. The element which has to be removed from the list will have an index that basically represents the 'index' in the syntax.
- It is not mandatory to pass an argument to the list in case there is no argument passed already. It will be comprehended as no argument passed on as -1, and by default, the last element will be popped out of the list. -1 is the default value which is the last place of the element in the list.
- The pop() method will return a list without that element that is mentioned to pop out using the method above. So basically, it returns the complete list except for the popped element.
- If the user specifies an index that is not in range, then the pop() method will throw an IndexError exception: pop index out of range Exception". Hence pop() function will stop working when the index is not in range.
- The following processes take place at the backend of the pop() method, which is updating the index, removing the item from the index, list reversal, etc.
Python List pop() Example
As we have already discussed before, the pop() function eliminates the items at the particular index and returns the list without the popped elements. After removing them, the elements which are left move up and fill the gap in the index. If the user is aware of the Index value and he wants to check the deleted ones, he can simply use this method to see them.
Below is a python code to understand how pop() function works in python:
x = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
print(x)
y = x.pop(1)
print(y)
print(x)
x.pop(2)
print(x)
Output:
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
2
[1, 3, 4, 5, 6, 7, 8, 9, 10]
[1, 3, 5, 6, 7, 8, 9, 10]
>
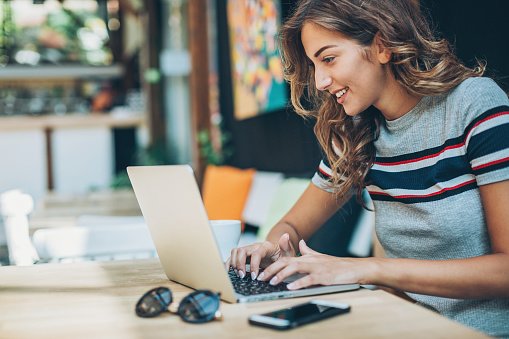
Python Training Certification
- Master Your Craft
- Lifetime LMS & Faculty Access
- 24/7 online expert support
- Real-world & Project Based Learning
Removing a Non-Existent Value with pop()
The python code will throw an exception if the user tries to eliminate an element that does not exist. This happens as the pop() function is unable to locate the element to which the user is trying to refer to. Hence, the pop() function, therefore, is not able to return any value.
Below is a python code to display the popped out element using the pop() function:
ruits = ['Apple', 'Orange', 'Kiwi', 'Cherry', 'Banana']
remove_fruit = fruits.pop(4)
print(remove_fruit)
Output:
Banana
Let us see another python code where the item is not present in the list which is being popped out by the user:
fruits = ['Apple', 'Orange', 'Kiwi', 'Cherry', 'Banana']
remove_fruit = fruits.pop(7)
print(remove_fruit)
Output:
Traceback (most recent call last):
File "", line 2, in
IndexError: pop index out of range
>
Python Dictionary pop()
The method Python Dictionary pop() aims to remove the (key, value) pair and return the desired value. However, in a case where the key is not present, there is a default argument that gets passed, and the pop() method returns the default value automatically. When the default value is not passed, the pop() method will throw an exception called the 'KeyError' Exception.
The syntax for writing python dictionary pop() is written below:
dict.pop(key[, default])
Here,
key is required to remove the duplicate key
default returns the value if no argument is not specified
Below is a python code to understand how python dictionary pop(key) method works:
dictionary = {'x': 10, 'y': 20, 'z': 30}
val = dictionary.pop('y')
print(val)
print(dictionary)
Output:
20
{'x': 10, 'z': 30}
>
In the above python code, we can observe that the user has passed key ‘y’ in the form of an argument to the pop() method. Hence, pop() returns the value and it eliminates the key:value pair from the dictionary.
Below is a python code to understand how the python dictionary pop(key, default) method works:
dictionary = {'x': 10, 'y': 20, 'z': 30}
val = dictionary.pop('u', 0)
print(val)
print(dictionary)
Output:
0
{'x': 10, 'y': 20, 'z': 30}
>
In the above code, we can observe that the key ‘u’ which the user has passed, in the form of an argument to pop() method is unavailable in the dictionary. Hence, the pop() method will return the default value that was passed as the 2nd argument. In this way, the dictionary will remain unchanged.
Want to Become a Master in Python Django? Then visit HKR to Learn Python Django Training!
Subscribe to our YouTube channel to get new updates..!
What Value Does Dictionary Pop In Python Return?
Let us see the key points below along with their examples and understand what value does a dictionary actually return:
1. Using dictionary pop() method when key is present
fruits = {'Apple': 1, 'Banana': 2, 'Cherry': 3, 'Kiwi': 4, 'Orange': 5}
print(fruits)
print ("The key value is:", fruits.pop('Cherry'))
print('The updated dictionary will be:', fruits)
Output:
{'Apple': 1, 'Banana': 2, 'Cherry': 3, 'Kiwi': 4, 'Orange': 5}
The key value is: 3
The updated dictionary will be: {'Apple': 1, 'Banana': 2, 'Kiwi': 4, 'Orange': 5}
>
2. Using dictionary pop() method when default value is present
fruits = {'Apple': 1, 'Banana': 2, 'Cherry': 3, 'Kiwi': 4, 'Orange': 5}
print(fruits)
a = fruits.pop('Grapes', 'Pear')
print('The popped element is:', a)
print('The dictionary is:', fruits)
Output:
{'Apple': 1, 'Banana': 2, 'Cherry': 3, 'Kiwi': 4, 'Orange': 5}
The popped element is: Pear
The dictionary is: {'Apple': 1, 'Banana': 2, 'Cherry': 3, 'Kiwi': 4, 'Orange': 5}
>
3. Using dictionary pop() method to understand Keyerror exception
fruits = {'Apple': 1, 'Banana': 2, 'Cherry': 3, 'Kiwi': 4, 'Orange': 5}
print(fruits)
print(fruits.pop('Pear'))
Output:
{'Apple': 1, 'Banana': 2, 'Cherry': 3, 'Kiwi': 4, 'Orange': 5}
Traceback (most recent call last):
File "", line 3, in
KeyError: 'Pear'
>
Become a saltstack Certified professional by learning this HKR Saltstack Training!
Python Set pop()
Python pop() method will aim at removing an arbitrary element from a set and will further return the removed element. The set Pop() method doesn't actually take a parameter. Below is the syntax for using set pop() method
set.pop()
Let us see an example below to understand how set Pop() method works in python:
a ={"Welcoming", 1, 2, 3, "you"}
print("The original Set:",a)
print("The removed element:",a.pop())
print("Set after pop():", a)
Output:
The original Set: {1, 2, 3, 'you', 'Welcoming'}
The removed element: 1
Set after pop(): {2, 3, 'you', 'Welcoming'}
>
Conclusion:
In this article, we have understood how the Pop() method is used inside a function in a python list. The article has also explained how pop works with a list, a dictionary and a non-existential value in python, python set pop() along with the return value of this method.
Python Pop FAQ’s
1. What does pop () do in Python?
Pop () method is actually a built-in python function that removes the element from the specified index in a list.
2. Is Python list pop thread-safe?
Yes, it is safe to use python list pop threads as they are atomic, and they won't change on coming up with a new thread.
3. How do I use pop in Python 3?
There is no different way of using pop in python 3. The user can use the pop() method in the same way as python.
4. What is the pop index out of range?
If the user specifies an index which is not in range, then the pop() method will throw an IndexError exception: pop index out of range exception". Hence pop() function will stop working when the index is not in range.
5. How is pop different from pop items?
Using the pop() method, the user can remove any item from a dictionary until the key is specified by the user. Whereas pop item() only removes the value and returns the last item from the dictionary.
6. How many arguments can pop take?
Pop () can take almost two parameters at a time. The defined answer will be either one or two.
7. How do you use pop?
As the user mentions the index to be removed, the value is popped out. However, when the index is not present, the last element is eliminated from the list by default.
Related Article
2. Python Ogre
About Author
As a content writer at HKR trainings, I deliver content on various technologies. I hold my graduation degree in Information technology. I am passionate about helping people understand technology-related content through my easily digestible content. My writings include Data Science, Machine Learning, Artificial Intelligence, Python, Salesforce, Servicenow and etc.
Upcoming Python Training Certification Online classes
Batch starts on 20th Apr 2025 |
|
||
Batch starts on 24th Apr 2025 |
|
||
Batch starts on 28th Apr 2025 |
|