Enumerate () in Python - Table of Content
- Introduction to Enumerate () in Python with an example
- Why python enumerate () is so important?
- Python Enumerate () function lists in detail
- Creating Python Dictionaries by using Enumerator functions
- Enumerate functions of a Python class
- Python Enumerate over an enum in C++
- ChainMap in Python
- Multiprocessing in Python
- Conclusion
Introduction to Enumerate ()in Python with an example
Enumerator () term commonly used in mathematical concepts and computer language to ordering the lists of all set elements. Python Enumerates () is built-in functions used in the form of indexes. When you are working with Iterations, Enumerators’ help them to keep tracking of the items in the iterator functions. Enumerate () functions contain tulips and also add the counter to the iteration.This enumerator function returns the output value in the object form.
The syntax is as follows;
Enumerate (iterable, start = 0)
Where iterable is a sequence, an iterator or objects that support iterations
The start is the position of the iteration sequences
The default is 0.
Become a python Certified professional by learning this HKR Python Training !
Example:
months = {‘Jan’, ‘Feb’, ‘Mar’, ‘Apr’}
enum_months = enumerate (months)
Print (type (enum_months))
Or
Print (list (enum_months)) # returns the days in list form
Enum_months = enumerate (months, 5) # count the months starting from 0
Print (list (enum_months))
Output:
[(0, ‘Jan’), (1, ‘Feb’), (2, ‘Mar’), (3, ‘Apr’)]
Or
[(5, ‘Jan’), (6, ‘Feb’), (7, ‘Mar’), (8,’Apr’)]
Why python enumerate () is so important?
There are a lot of advantages that make Enumerate () function so important while working with the python programming language. As I said earlier,with Enumerate () function users can easily list all the items. I would like to mention few importance of using Enumerate ();
- When users working with iteration, enumerate () keep tracking of all the iteration cells.
- Enumerate () is a built-in function that adds counter to any iteration methods.
- Enumerate () object can be used directly in loops and converted them into tulip lists.
- The enumerate () function is complete and easy to add indexes to any loops.
- This function makes the user debug easily and write codes with fewer errors.
Python Enumerate () function lists in detail
Python enumerates () functions are nothing but a set of rules and procedures to perform specific operations or tasks. Let me note a few major functions of Enumerate () in python;
Abs () -> this function returns the absolute value of a number.
All () -> this returns True if all values in an iterable object are true
Any () -> this returns True if any values in the objects are true
ASCII () -> returns only special characters in the list, and also this replaces the none-ASCII special characters with escape types.
Bin () -> returns the binary version of a number
Bool () -> Returns the Boolean value of the specified object
Bytearray () -> returns an array of bytes
Bytes () -> returns byte of an object
Callable () -> returns true if the specified object is callable, otherwise false
Chr () -> returns a character from the specified Unicode
Classmethod () ->converts method into a class method
Compile () -> returns the specified source as an object, ready to be executed
Complex () -> returns a complex number
Exec () -> executes the specified code (or object)
Filter () -> use a filter function to exclude items in an iterable object
Getattr () -> returns the value of the specified attributes (property or method)
Range () -> returns a sequence of numbers, starting from 0 and increments by 1 (by default)
Super () -> returns the object that represents the parent class
Tuple () -> returns a tuple.
Creating Python Dictionaries by using Enumerator functions:
Here Python enumerates () function accepts the iterable values as an argument and returns them as an object.This contains an index and corresponding iterable objects as lists,tulips,and strings.
Then these enumerate objects which store them as an index and value converted into a dictionary using python dictionary comprehensions.
Example with code is as follows:
Var12 = [‘AA’, ‘BB’, ‘CC’, ‘DD’]
enum = enumerate (Var12)
enum;
d = dict ((i, j) for i,j in enum)
d;
Output is as follows:
{0: ‘AA’, 1: ‘BB’, 2: ‘CC’, 3: ‘DD’}
Enumerate functions of a Python class
The following code list print the function of the given class is as follows;
Class fun
def _ init_ (self):
self. x = x
def bar (self)
Pass
def baz (self):
Pass
Print (type (fun))
Import inspect
Print (inspect. getmembers (fun, predicate = inspect. ismethod))
Output is as follows:
[ (‘ __init__’,), (‘bar’, ), (‘baz’, )]
Python Enumerate over an enum in C++
Enumerate () function is used as a data type in C or C++ programming language. This data type is used to assign names to the integral constraints and makes the program easier to run.
The keyword enum is used to declare enumeration variables.
The syntax is as follows;
Enum enum_name {const1, const2…};
Where enum_name indicates the namespace of a variable
Const1 and const2 are the types of name flags
Enum also defines the type of enum data variables.
We can define enum in C using two ways;
Enum color_name {black, white};
Enum suit_name {heart, diamond = 7, spade =2, cub};
Example
#include
Using namespace fun;
enum color_name {black=7, white};
enum suite_name {heart, diamond =7, spade =2, club};
int main ()
{
Count Count Return 0;
}
Output:
The value of enum color_name: 7, 8
The default value of enum suit_name: 0, 7, 2, 3
Now come to enumerate over an Enum in C/C++. This is like an essay process, here we need to create a loop and will start from the first default type = 0. Let's see the code;
#include
Using namespace fun;
Enum suit_name {heart, diamond, spade, club};
Int main ()
{
For (int i = heart; i {
Count }
}
Output:
Card type: 0
Card type: 1
Card type: 2
Card type: 3
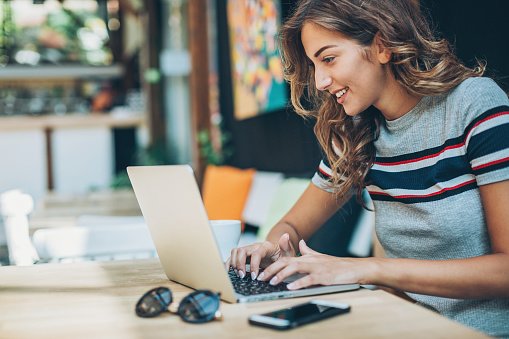
Python Training Certification
- Master Your Craft
- Lifetime LMS & Faculty Access
- 24/7 online expert support
- Real-world & Project Based Learning
ChainMap in Python
The ChainMap is used to encapsulate the dictionaries in Python into a single unit. It is a standard library which is located in the collections Module. Before using this ChainMap, we need to import it into the collection standard library module.
import collections
Here we use three types of functions such as maps (), keys (), and values (). Let’s see one by one with examples.
Map () function is used to display all the key-value pairs in ChainMap from the dictionaries.
Keys () function is used to returns the key value from the ChainMap.
Values () function is used to return values () of different keys () method from the ChainMap.
Example:
Import collections as fun
Con_code1 = {‘India’: ‘IN’, ‘America’: ‘USA’}
Con_code2 = {‘Africa’: ‘SA’, ‘Australia’: ‘AUS’}
Chain = fun. ChainMap1 (con_code1, con_code2)
Print (“first chain: “+ str (chain.maps1))
Print (“the key values in the ChainMap1: “ + str (list (chain. Keys ())))
Print (“the values in the ChainMap1: “ + str (list (chain. Values ())))
Output:
First chain: [{‘India’: ‘IN’, ‘America’: ‘USA’}, {‘Africa’: ‘SA’, ‘Australia’: ‘AUS’}]
The keys in the ChainMap : [‘America’, ‘Australia’, ‘India’, ‘Africa’]
The values in the ChainMap: [‘USA’, ‘AUS’, ‘IN’, ‘SA’]
Top 50 frequently asked Python interview Question and answers !
The new_child () method and reversed method:
The new_child () method is used to add another new python dictionary object to the ChainMap at the initial step. The reversed method is used to reverse the order of ChainMap key_value pairs.
Example:
Import collections as fun
Con_code1 = {‘India’ : ‘IN’, ‘America’ : ‘USA’}
Con_code2 = {‘Africa’: ‘SA’, ‘ Australia’: “AUS’}
Newcode = {‘Russia’: ‘RUS’}
Chain = col.chainmap1 (con_code1, con_code2)
Print (“First chain: “ + str (chain. Maps))
Chain = chain. New_child (Newcode) # insertion of new child
Print ("chain after insertion of the new child: “ + str (chain. Map))
Chain. Maps = reversed (chain. Maps)
Print (“Reversed chain value: “ + str (chain))
Output:
Initial chain: [{‘India’ : ‘IN’, ‘America’ : ‘USA’}, {‘Africa’ : ‘SA’, ‘Australia’ : ‘AUS’ }]
Chain after inserting new child: [{‘Russia’ : ‘RUS’}, {‘India’ : ‘IN’, ‘America’ : ‘USA’}, {‘Africa’ : ‘SA’, ‘Australia’ : ‘AUS’}]
Reversed chain value : ChainMap({ ‘Africa’ : ‘SA’, ‘Australia’ : ‘AUS’}, {‘India’ : ‘In’, ‘America’ : ‘USA’}, {‘Russia’:’RUS’})
NamedTuped in Python:
namedtuple is also a type of method that comes under Collection module. These contain keys that are mapped to values. Here we access all the elements using keys and indexes.
The standard syntax is as follows:
Import collections.
In Namedtuple method in python uses the getattr () function, which is used to get the data object form the attributes.
Example:
Import collections as fun
Employee = fun. Namedtuple (‘employee’, [‘name’, ‘city’, ‘salary’]) # creation of employee Namedtuple.
Emp1 = employee (‘Bavya’, ‘Bangalore’, ’50000’)
Emp2 = employee (‘ram’, ‘Delhi’, ’25000’)
Print (‘the name and salary of emp1 : ‘ + emp1 [0] + ‘and ‘ + emp1 [2])
Print (‘the name and salary of emp2 : ‘ + emp2.name + ‘and’ + emp2.salary)
Print (‘the city of emp1 and emp2 : ‘ + getattr(emp1, ‘city’) + ‘and’ +getattr (emp2, ‘city’))
Output:
The name and salary of emp1: Bavya and 50000
The name and salary of emp2: Ram and 25000
The city of emp1 and emp2: Bangalore and Delhi
Conversion procedure of Namedtuple:
There are some methods in the python, which need to be converted into Namedtuple. Here we use the _make () method to convert any iterational objects like list, tuple, etc. to Namedtuple object.
Example:
Import collections as fun
Employee = fun. namedtuple (‘Employee’, [‘name’, ‘city’, ‘salary’])
My_list1 = [‘Bavya’, ‘Bangalore’, ‘50000’]
Emp1 = employee_make (my_list1)
Print (emp1)
My_dict1 = {‘name’: ‘Ram’, ‘city’ : ‘Delhi’, ‘Salary’ : ‘25000’}
Emp2 = employee (**my_dict1)
Print (emp2)
Emp_dict = e1._asdict1 ()
Print (emp_dict1)
Output:
Employee (name = ‘bavya’, city = ‘Bangalore’, salary = ‘50000’)
Employee (name = ‘Ram’, city = ‘Delhi’, salary = ‘25000’)
OrderedDict ([(‘name’, ‘Bavya’ ), (‘city’, ‘Bangalore’), (‘Salary’, ‘50000’)])
Deque in Python:
Deque in python is nothing generalization of queue structure or stack, here it should be initialized from left to right. In python, we should use, list objects to create a deque. This offers 0 (1) complexity in the popping and appending.
Deque is also available in the form class library located in the Collection module.
The syntax is as follows;
Import collections
1. The appending functions on Deque:
There are two types of append available;
a) append () this method is used to add/insert the elements from the right-hand side of the queue.
b) appendleft () this method is used to add/insert the elements from the left-hand side of the Queue.
Example:
Import collections as fun
My_deque1 = fun. Deque (‘234abcd’)
Print (‘dequeue: ‘ + str (my_deque))
My_deque1.append (‘x’)
My_deque1.appendleft (‘A’)
Print (‘Dequeue after appending: ‘ + str (my_deque1))
Output:
Dequeue : deque ([‘2’, ‘3’, ‘4’, ‘a’, ‘b’, ‘c’,‘d’])
Dequeue after appending: deque ([‘A’, ‘2’, ‘3’, ‘4’, ‘a’, ‘b’, ‘c’, ‘d’,’ X’])
Output:
Dequeue : deque ([‘2’, ‘3’, ‘4’, ‘a’, ‘b’, ‘c’,‘d’])
Deque after appending: ([‘A’, ‘2’, ‘3’, ‘4’, ‘a’, ‘b’, ‘c’, ‘d’ ‘x’])
The popping function on Deque:
Like append function here also there are two methods such as pop () is used to remove/delete and return only rightmost elements and the popleft () method is used to remove/delete and return only left-most elements.
Example:
Import collections as fun
My_deque1 = fun.deque (‘234abcd’)
Print (‘Dequeue: ‘ + str (my_deque1))
Item1 = my_deque1.pop ()
Print ( ‘ popped item: ‘ + str (item))
Item2 = my_deque1.popleft ()
Print ( ‘popped item: ‘ + str (item))
Print (‘ Dequeue after pop operations: ‘ + str (my_deque1))
Output:
Dequeue: deque ([ ‘2’, ‘3’, ‘4’, ‘a’, ‘b’, ‘c’, ‘d’])
Pop item : d
Popped item: 2
Dequeue after pop operations: deque ([‘3’, ‘4’, ‘a’, ‘b’, ‘c’, ‘d’ ])
OrderedDict in Python:
OrderedDict in python is a subclass dictionary object. The main difference between OrderedDict and normal Dict is that in OrderedDict we can order the inserted key values in a list and where is in Dict, the ordering may not happen or not in most of the cases.
This OrderedDict is also located in the Collections module.
The syntax is as follows:
Import collections
Example:
Import collections
My_dict1 = {}
My_dict1 [‘aa’] = 11
My_dict1 [‘bb’] = 22
My_dict1 [‘cc’] = 33
For item in my_dict1.items ():
Print (item)
Print ()
My_ord_dict1 = collections. OrderedDict ()
My_ord_dict1 [‘aa’] = 11
My_ord_dict1 [‘bb’] = 22
My_ord_dict1 [‘cc’] = 33
For item in my_ord_dict1. Item ():
Print (item)
# Dict Output:
(‘aa’, 11)
(‘bb’, 22)
(‘cc’, 33)
#OrderedDict output:
(‘aa’, 11)
(‘bb’, 22)
(‘cc’, 33)

Subscribe to our YouTube channel to get new updates..!
Changing the value of a specified key:
Here we can change the key values only with Dict objects in Python. But we can’t change the specified key values with OrderedDict objects. Let see the example that explains it;
Example:
Import collections
My_dict1 = {}
My_dict1 [‘aa’] = 11
My_dict1 [‘bb’] = 22
My_dict1 [‘cc’] = 33
For item in my_dict1.items ():
Print (item)
My_dict1 [‘bb’] = 200
Print (‘after change key value in dict’)
For item in my_dcit1. Items ():
Print (item)
Print ()
My_ord_dict1 = collections. OrderedDict ()
My_ord_dict1 [‘aa’] = 11
My_ord_dict1 [‘bb’] = 22
My_ord_dict1 [‘cc’] = 33
For item in my_ord_dict1. Items ():
Print (item)
My_ord_dict1 [‘bb’] = 200
Print ('after changing the value in ordered dict’)
For item in my_ord_dict1. Items ():
Print (item)
Output:
(‘aa’, 11)
(‘bb’, 22)
(‘cc’, 33)
After changing key values in Dict:
(‘aa’, 11)
(‘bb’, 22)
(‘cc’, 33)
(‘bb’, 200)
(‘aa’, 11)
(‘bb’, 22)
(‘cc’, 33)
After changing in Ordered Dict:
(‘aa’, 11)
(‘bb’, 200)
(‘cc’, 33)
Become a Magento Certified professional by learning this HKR Magento Training !
Multiprocessing in Python:
The multiprocessing in python supports the spawning of multiple packages. This multiprocess function refers to the loading and execution of a new child process. To terminate or perform computing operations, they make use of API threading.
To execute the multiprocess function, first, we should create process objects. Then we call it a start () method.
Example:
From multiprocessing import process
def display ():
Print (‘hello world)
If _ name _ == ‘__main__’:
P = process (target = display)
P. start ()
P. join ()
Case1: we can also pass a parameter into the functions using Arguments (args) keyword as shown in the below example.
From multiprocessing import process1
Def display (my_name1):
Print (‘Hello!!! ‘ + “ “ + my_name1)
If _name__ == ‘ __main__’:
P = process (target = display, args = (‘python’,))
P. start ()
P. join ()
Case 2: in the following example we can create the function that calculates the number of cube and point.
From multiprocessing import process
Def cube1 (X):
For X in my_numbers1:
Print (‘%s cube is %s’ % (X, X*3))
If _name__ == ‘__main__’:
My_numbers1 = [3, 4, 5, 6, 7, 8]
P = process (target = cube1, args = (‘X’, ))
P. start ()
P. join
Print ( “done”)
Output:
3 cube1 is 27
4 cube1 is 64
5 cube1 is 125
6 cube1 is 216
7 cube1 is 343
8 cube1 is 512
Case 3: we can also create more than one process at a time, in the below example code explains it.
From multiprocessing imports process
Def cube1 (x):
For x in my_numbers1:
Print (‘%s cube1 is %s’ % (x, x**3))
Def evenno1 (x):
For x in my_numbers1:
If x % 2 == 0:
print ( ‘%s is an even number1 ‘ % (x))
If __name__ == ‘ __main__’:
My_numbers1 = [3, 4, 5, 6, 7, 8]
My_process1 = process1 (target1 = cube1, args = (‘x’, ))
My_process2 = process1 (target1 = evenno, args = (‘x’,))
My_process1.start ()
My_process2.start ()
My_proces1.join ()
My_process2.join ()
Print (“Done”)
Output:
3 cube1 is 27
4 cube1 is 64
5 cube1 is 125
6 cube1 is 216
7 cube1 is 343
8 cube1 is 512
4 is an even number
6 is an even number
8 is an even number
Done
Quine in Python:
Quine is a special type of function, which doesn't take any input but also produces the output. The mandatory condition here we should follow is that we cannot open the source code files inside the included program.
Example:
a = ‘a = %r; print (%a %a) ‘ ; print (a%a)
Output:
a= ‘a=%r; print (a%%a) ‘ ; print (a%a)
We can also perform some task using the following code is as follows;
Print (open (__file__). read ())
Conclusion
Enumerate () in python is a special type of function which is used to the ordering of the list in the form of indexes. This type of function is mainly used to add iterate into the lists.I hope this blog may help a few of you to learn Python in-depth and also mater the code concepts.
Related Articles:
About Author
As a senior Technical Content Writer for HKR Trainings, Gayathri has a good comprehension of the present technical innovations, which incorporates perspectives like Business Intelligence and Analytics. She conveys advanced technical ideas precisely and vividly, as conceivable to the target group, guaranteeing that the content is available to clients. She writes qualitative content in the field of Data Warehousing & ETL, Big Data Analytics, and ERP Tools. Connect me on LinkedIn.
Upcoming Python Training Certification Online classes
Batch starts on 25th Dec 2024 |
|
||
Batch starts on 29th Dec 2024 |
|
||
Batch starts on 2nd Jan 2025 |
|