What is a list in python?
A list is a data structure that contains an ordered sequence of elements.It is a mutable or changeable object. The values in the list are called items.The values can be of different types.We can gather related set of values together that belong to a group and store them in a list. It enables us to perform any operation on all the data present in a list at once.The items should be enclosed in square brackets and separated by a comma (,). We can create a list with a combination of numbers and string characters. A list can be defined in the following ways.
ListA = [1, 2, 3, 4];
ListB = ['Hi', 'Hello'];
ListC = ["Hi", "Hello"];
Become a python Certified professional by learning this HKR Python Training !
Find the length of a list
The length of a list in python can be found through two methods.
Len() Method
It is a built-in function provided by python for getting the total number of items in a list, array, dictionary, etc. It takes the name of the list as an argument and returns the length of the given list. The len() method is the most commonly used method in python for finding the length of a list. Here is the syntax of the length method.
len(
Let's look at an example program.
ListA = [1, 2, 3, 4, "Hello", "World"]
print ("Number of items in the list = ", len(ListA))
The output will be as follows.
Number of items in the list = 6
We can also give the list directly as an argument to the len() method.
Length = len([1, 2, 3, 4, "Hello", "World"]);
print("Number of items in the list = ", Length);
The output will be as follows.
Number of items in the list = 6
Length_hint() method
This method is not much known to programmers. It takes in a list or list name as an input argument and returns the number of items in the list. Here is a sample program that shows the use of the length_hint() method.
from operator import length_hint
ListA = [1, 2, 3, 4, "Hello", "World"]
print ("Number of items in the list = ", length_hint(ListA))
The output for the above program is as follows.
Number of items in the list = 6
Naive Method
Apart from the len() method, there is another way to find the length of a list in Python. The naive approach is one of the most common methods when there is no alternative. It allows you to run the loop and count the items. Let us see its implementation :-
ListA = [1, 2, 3, 4, "Hello", "World"];
print("Input List = ", str(ListA));
count = 0;
for i in ListA:
count = count + 1
print("Number of items in the list = ", count);
The output for the above program will be as follows.
Input List = [1, 2, 3, 4, 'Hello', 'World']
Number of items in the list = 6
This is the most basic method for finding the length of a list. But the len() method is always preferable to use.
Acquire Keras certification by enrolling in the HKR Keras Training program in Hyderabad!
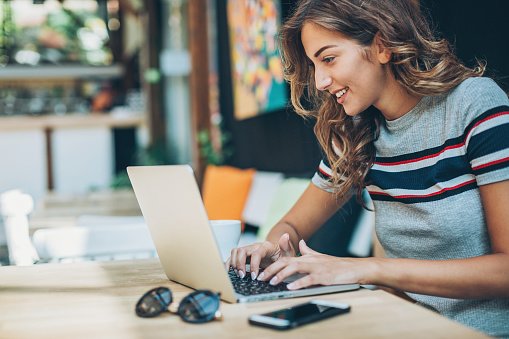
Python Training Certification
- Master Your Craft
- Lifetime LMS & Faculty Access
- 24/7 online expert support
- Real-world & Project Based Learning
Performance analysis of the three methods
Before choosing a method, we can do performance analysis and choose based on that. Let's take a sample program that calculates the time taken to run each method.
from operator import length_hint
import time
ListA = [1, 2, 3, 4, "Hello", "World"];
print("Input List = ", str(ListA));
#Navie method
naive_start = time.time()
count = 0
for i in ListA:
count = count + 1
naive_end = str(time.time() - naive_start)
print ("Time taken using naive method = ", naive_end)
# len() method
len_start = time.time()
list_len = len(ListA)
len_end = str(time.time() - len_start)
print ("Time taken using len() method = ", len_end)
# length_hint() method
hint_start = time.time()
list_len_hint = length_hint(ListA)
hint_end = str(time.time() - hint_start)
print ("Time taken using length_hint() method = ", hint_end)
The output for the above program will be as follows.
Input List = [1, 2, 3, 4, 'Hello', 'World']
Time taken using naive method = 4.76837158203125e-07
Time taken using len() method = 1.6689300537109375e-06
Time taken using length_hint() method = 9.5367431640625e-07
We can see that the len() method has taken less time than the other methods.

Subscribe to our YouTube channel to get new updates..!
Using length method in a loop
The len() method not only returns the length of the list, but it can also return the length of each string in the list. We can loop through the list, apply the len() method on them to get the number of characters of an item specified within the list. We can only apply it on the string items given in the list. Let's see how we can do that.
ListA = ["Hello", "World", "Welcome", 3, 4]
print ("Number of items in the list = ", len(ListA))
for i in ListA:
print("Length of item = ", len(i))
Top 50 frequently asked Python interview Question and answers !
The output for the above program will be as follows.
Number of items in the list = 5
Length of item = 5
Length of item = 5
Length of item = 7
As you can see here, it only returned the length of only string items.
Checking whether a list is empty or not
We can check if a list is empty or not in our python program using the len() method. Based on the return value of the len() method, we can specify a set of actions to be performed. Let’s take a look at a sample program of it.
ListA = ["Hello", "World", "Welcome", 3, 4]
ListB = []
print ("Number of items in ListA = ", len(ListA))
print ("Number of items in ListB = ", len(ListB))
if len(ListA) == 0:
print("The ListA is empty")
else:
print("The ListA is not empty")
if len(ListB) == 0:
print("The ListB is empty")
else:
print("The ListB is not empty")
The output for the above program will be as follows.
Number of items in ListA = 5
Number of items in ListB = 0
The ListA is not empty
The ListB is empty
Conclusion
The len() is the method used by all the programmers today.Now that you know the utility of the len() method, you can use it in various ways, as explained in this post. Start experimenting with finding the length of a list in your code. For more information on python,refer to the python tutorials and blogs on our website.
Related Articles:
About Author
As a senior Technical Content Writer for HKR Trainings, Gayathri has a good comprehension of the present technical innovations, which incorporates perspectives like Business Intelligence and Analytics. She conveys advanced technical ideas precisely and vividly, as conceivable to the target group, guaranteeing that the content is available to clients. She writes qualitative content in the field of Data Warehousing & ETL, Big Data Analytics, and ERP Tools. Connect me on LinkedIn.
Upcoming Python Training Certification Online classes
Batch starts on 22nd Jun 2025 |
|
||
Batch starts on 26th Jun 2025 |
|
||
Batch starts on 30th Jun 2025 |
|