Python Flask Tutorial - Table of Content
- Python flask
- Advantages of Python flask
- What are APIs?
- Creating your first flask application
- Creating an API
- Conclusion
Python flask
Flask is a lightweight web application framework provided by python. A web application framework represents a collection of libraries that help in building web applications quickly. The web applications can be of web pages, or it can range to complex commercial applications. It does not have a database abstraction layer. It is developed by Armin Ronacher of the ‘Pocoo’ and released initially on April 1st, 2010. It initially began as a wrapper around Werkzeug and Jinja projects.
Werkzeug - It is a WSGI (web server gateway interface) utility from python.It is an interface between the web server and the web applications. It provides useful functions and classes for the WSGI application.
Jinja - It is a templating language for python. It comes with an optional sandboxed template execution environment.It is similar to the Django template. We can create HTML, XML, or other markup formats.
The latest stable version (1.1.2) release of python flask is on April 3rd, 2020.It is voted as the most popular web framework in the Python Developers Survey 2018.It has a lot of stars on GitHub than Django and other python web application frameworks. Flask doesn't enforce any dependencies.
Become a Python Certified professional by learning this HKR Python Training !
Advantages of Python flask
Flask offers a variety of benefits, that's why the developers like to work with Python flask. Let's look at some of the benefits of the flask.
- Flask is easy to use and read. So the developers can easily understand the code written by other programmers.
- It is a microframework and has a modular design, so it is easy to transit.
- It allows quick prototyping.
- We can scale up to creating complex web applications.
- It handles HTTP requests.
- It provides better performance.
- It has a built-in development server.
- It provides support for secure cookies.
- We will have more control over the application development.
- It supports integrated unit testing.
- It uses Jinja templating.
- We can plugin our favorite ORM.
- It supports a lot of extensions that we can add to any application features that we like.
- It is WSGI 1.0 compliant, so it is easier to deploy flask in production.
- We will have more flexibility in configuration.
What are APIs?
An API is short for Application Programming Interface. Computer programs have to communicate with other programs or with the underlying operating system. This is when we require APIs to establish communication. An API is a computer program that allows manipulating information by another program over the internet. We can share data with other users through APIs. Here are some keywords to remember while creating APIs.
HTTP (Hypertext Transfer Protocol) - It is the primary means of data communication over the web. It has methods that specify the movement of data direction.
URL (Uniform Resource Locator) - It represents the address of a resource on the web. It consists of a protocol, domain, and an optional path.
JSON (JavaScript Object Notation) - An API return data in the form of JSON. It is a data format that is easily readable by users and machines.
REST (REpresentational State Transfer) - It represents the best practices for implementing APIs.The APIs that follow REST principles are called REST APIs.
HTTP methods
HTTP request methods indicate the action to be performed on a resource. We have to specify which HTTP method should handle the request in the flask route function. Let's look at the different HTTP methods.
GET - It sends data in the form of unencrypted to the server. It is the most commonly used method. The flask route responds to GET requests by default. It only retrieves data from the user.
HEAD - It functions the same as the GET method but without a request body.
POST - We can use POST to send form data to the server. The server cannot cache the received data.
PUT - It replaces all the current representations of the target resource with the request payload.
DELETE - It deletes all the current representations of the target resource.
Top 30 frequently asked Python Interview Questions !
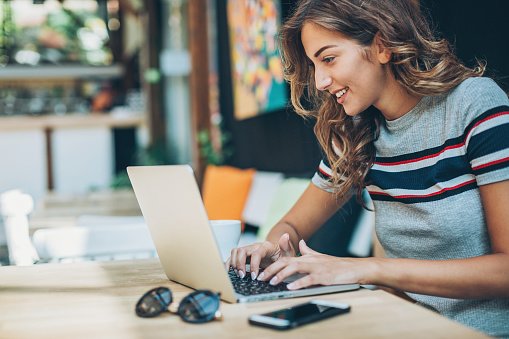
Python Training Certification
- Master Your Craft
- Lifetime LMS & Faculty Access
- 24/7 online expert support
- Real-world & Project Based Learning
Creating your first flask application
First, make sure that python is functional in your system. Open command prompt and type in python.You should be able to see the python version and other information. You will also be in an interactive prompt where you can execute python code. Let us create a simple flask application.
In python, all the application code will be present in a subdirectory and should include an ' __init__.py' file. The __init__.py file is a package that can be imported and executed. Create a project folder with 'SampleApp' as the name. Create a new file in your favorite text editor and paste the following code in it.
#Import flask module into the project
from flask import Flask
#Create a flask object
app = Flask(__name__)
#URL mapping of the associated function
@app.route('/')
#Specify the server response to return
def first_application():
return 'Welcome to the HKR Trainings'
#The main driver function
if __name__ == '__main__':
#Run the application
app.run()
You can find comments in the code that explains every line. Save the file with name as script.py. Open the command prompt and navigate to your project folder using the below command.
cd
Give the below command to run your application.
python script.py
You can see the logs of the application on the command line. It will show the URL on which the application is running too. The URL will usually be http://localhost:5000. Open a browser and run http://localhost:5000. You should be able to see a message that says 'Welcome to the HKR Trainings'.
Related Articles: Python operators !

Subscribe to our YouTube channel to get new updates..!
Creating an API
Now that you know how to create and run a python application. Let us create an API that can return some information. Let us add our training tutorials as a list of python dictionaries. Dictionaries in python will be of key-value pairs. Each dictionary should have a unique id through which it can be identified.
Create a new project with 'TrainingLinks' as a name. Open a new file in a text editor and paste the following code in it.
import flask from Flask
import request, jsonify
app = Flask(__name__)
app.config["DEBUG"] = True
tutorials = [
{'id': 0,
'Name': 'ELK Stack Tutorial',
'Link': 'https://hkrtrainings.com/elk-stack-tutorial'},
{'id': 1,
'Name': 'Tosca Tutorial',
'Link': 'https://hkrtrainings.com/tosca-tutorial'}
]
@app.route('/', methods=['GET'])
def home():
return 'Welcome to the HKR Trainings'
@app.route('/api/v1/hkr/tutorials', methods=['GET'])
def api_all():
return jsonify(tutorials)
app.run()
Run the application from your command prompt. Now when you open a browser and run http://localhost:5000. You should be able to see a message that says 'Welcome to the HKR Trainings'. When you navigate to http://localhost:5000/api/v1/hkr/tutorials, you can see the list of tutorials that we have included in the code.
[ Related Article: elk stack tutorial ]
Conclusion
Flask enhances the development of complex web applications. App routing is the most important part of python flask,as it maps the application to specific functions.We can create APIs for data present in files, databases, etc. Flask provides extensions for Mail,WTF, SQLite, SQLAlchemy,and many more.With flask,we can create web applications very easily without putting much effort.Companies like Netflix, Reddit, MailGui are using flask for building their web applications.
Related Articles:
About Author
As a senior Technical Content Writer for HKR Trainings, Gayathri has a good comprehension of the present technical innovations, which incorporates perspectives like Business Intelligence and Analytics. She conveys advanced technical ideas precisely and vividly, as conceivable to the target group, guaranteeing that the content is available to clients. She writes qualitative content in the field of Data Warehousing & ETL, Big Data Analytics, and ERP Tools. Connect me on LinkedIn.
Upcoming Python Training Certification Online classes
Batch starts on 20th Jun 2025 |
|
||
Batch starts on 24th Jun 2025 |
|
||
Batch starts on 28th Jun 2025 |
|