What are python scripts?
A python script is a collection of commands in a file. It is usually referred to as a python program stored in a file.It might contain function definitions and the main program that call functions.It is saved with a .py extension. When we want to run a set of tasks sequentially,we write them in a file and execute them.
Python interpreter
A python interpreter is an application that runs your script. It supports interactive editing,history substitution, etc. When you run a python script, some steps will be performed. It will start processing the commands in your script in a sequential fashion. It translates the Python code to bytecode, which is a lower-level language. Then the byte code is run over Python Virtual Machine (PVM). The PVM is a run time engine of python that iterates through the byte code and runs them one after the other.
Become a python Certified professional by learning this HKR Python Training !
Accessing the python prompt
To run a python script, you have to install python on your machine and make sure that it works. Let us see how we can run this python script from a command prompt on various operating systems.
Windows
Open command prompt by going to the Run box and type in 'cmd'. Navigate to the directory where Python is installed. Run the command 'python' on the command line. If python is working properly, you will be able to see the version of python. You will also be taken to the python prompt. To exit from this prompt, press 'ctrl + z'.
Mac
Open the launchpad and search for the terminal. When the terminal opens, type in python. You can see the version of the python as output. You will also be taken to the python prompt.
Linux
Right-click on Desktop and select terminal. Once the terminal opens, type python and run it. You will be able to see the python version as output.
If you have any doubts on Python, then get them clarified from Python Industry experts on our Python Community
Running python scripts from the command line
Let's take an example script and see how we can run it through the command line. Open a text file and copy the below code into it.
Name = "John"
print("Hello " + Name)
Save the file as hello.py format. Go to the command line and navigate to the folder where you stored hello.py file. Give the below command and click enter.
cd
python hello.py
The output will be as follows.
Hello John
Running python scripts in interactive mode
In interactive mode, we can write the script in a line-by-line manner. To enter into interactive mode, you just have to go to the python prompt. Give the below lines of code in the interactive mode.
Name = "John"
print("Hello " + Name)
When you press enter, you will be able to see the below output in the interactive mode.
Hello John
Let us also try running this sample program as well.
x = 5
y = 7
z = x+y
print("The value of x+y = ", z)
The output for the above script will be as follows.
The value of x+y = 12
Passing the command line arguments to python script
The command-line arguments help in passing inputs to your code. Whenever you want to read some data, you don't have it change it every time inside your script. You can just pass them as arguments while running the script. To achieve this, we have to use the sys built-in python module. Let's take an example and see how it works. Copy the below code to a text file and save it as ‘add.py’.
import sys
x = sys.argv[1]
y = 3
z = int(x) + y
print("The value of x+y = ", z)
In the command line, run the below command.
python add.py 10
The output for the above program will be as follows.
The value of x+y = 13
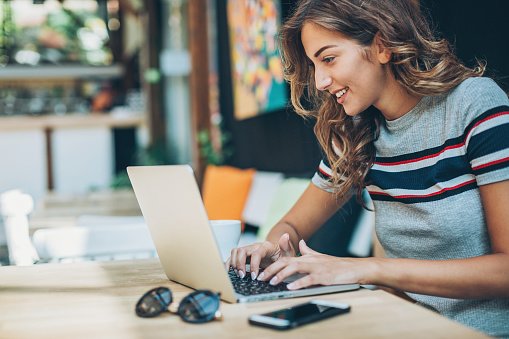
Python Training Certification
- Master Your Craft
- Lifetime LMS & Faculty Access
- 24/7 online expert support
- Real-world & Project Based Learning
Writing the output of python script to a file
We can save the output of a python script to a text file using the ‘>’ operator. Since you already have the add.py file, let us use the same python script. Give the below command in the command line.
python add.py 10 > output.txt
After you run the command, you won’t see anything on the command line. It will just go to the next prompt. Go to the folder where you have stored the add.py file. There you can see an output.txt file. Open the file and you can see the below output in it.
The value of x+y = 13
Running python scripts from the file manager
There is another simple way to run your python scripts. You can just navigate to the folder in which you have stored the add.py file and double-click on it. This is not a recommended way of running a python script.
To run the python script with a double-click, the file must have the execution permissions in Unix systems. To enable this feature in Windows, associate the .py and .pyw extensions with the python.exe and pythonw.exe programs respectively.
Running python scripts from an IDE
Name = "John"
print("Hello " + Name)
Right-click on the editor text area and select the 'Run File in Python Console' option. A console box will open at the bottom of the IDE, and it will show the below output.
Hello John
Subscribe to our YouTube channel to get new updates..!
Running python scripts from a text editor
To run a python script from a text editor like VS Code (Visual Studio Code), we have to add the python extension. Open the VS Code and go to the extension section. Search for 'python' and 'code runner' extensions and install them. Restart your VS Code to get the change into effect. Click on 'File' from the top menu and click on 'New File'. Give the name as 'hello.py'. Copy the below code in it and save the file.
Name = "John"
print("Hello " + Name)
Right-click on the editor text area and select the 'Run Code' option. A console box will open at the bottom of the IDE, and it will show the below output.
Hello John
Top 50 frequently asked Python interview Question and answers !
Running python scripts from a Notebook
The notebook is similar to an IDE and we can run our python scripts in it. Open Jupyter Notebooks, click on 'New' on the top menu. Select the 'Python File' option. It will create a new file and you will be at the user input line. Copy the below code in it.
Name = "John"
print("Hello " + Name)
Save the file by pressing 'ctrl + s'. Unlike other ways, the file will be saved with a '.ipynb' extension. Click on the ‘Run’ button, and you can see the below output in the console on the bottom right corner.
Hello John
Conclusion
Getting to know about running python scripts will be a building block to your python coding career.These will make your fundamentals strong and the development process much faster.Now that you know how to run the python scripts, pick your favorite way, and start building your applications in python code. For more information on python, refer to the python tutorials and blogs on our website.
Related Articles:
About Author
As a senior Technical Content Writer for HKR Trainings, Gayathri has a good comprehension of the present technical innovations, which incorporates perspectives like Business Intelligence and Analytics. She conveys advanced technical ideas precisely and vividly, as conceivable to the target group, guaranteeing that the content is available to clients. She writes qualitative content in the field of Data Warehousing & ETL, Big Data Analytics, and ERP Tools. Connect me on LinkedIn.
Upcoming Python Training Certification Online classes
Batch starts on 9th Jul 2025 |
|
||
Batch starts on 13th Jul 2025 |
|
||
Batch starts on 17th Jul 2025 |
|