Python Interview Questions
Last updated on Nov 21, 2023
Python is a high-level, interpreted, general-purpose programming language. Python can build any type of applications with its available tools/libraries. Python helps in modelling real-world problems and builds the applications to solve these issues. It supports objects, modules, threads, exception-handling and automatic memory management.
In this article, you can go through the set of python interview questions most frequently asked in the interview panel. This will help you crack the interview as the topmost industry experts curate these at HKR trainings.
Let us have a quick review of the python interview questions.
Most Frequently Asked Python Interview Questions
What are the local variables and global variables in python?
Ans:
Local Variables: The variables which are declared inside a function is known as a local variable. The scope of a variable is present in local space but not in global space.
Global Variables: The variables which are declared outside a function or in a global space are known as global variables. The scope of these variables is accessible by any function in the program.
Is indentation required in Python?
Ans: Indentation is mandatory in python. The indentation specifies the block of code.The loops, classes,functions etc are specified with indentation to represent it as a code of an indented block. The indentation is provided with four space characters.If the code is not indented it may not execute accurately and throws an error.
Wish to make a career in the world of Python? Start with Python Training !
How do you copy an object in python?
Ans: Unlike other programming codes, assignment “=” operator in python is not used in copying the objects; instead it creates a binding between the existing object and the target variable name. There are two ways of creating copies for the given object using the copy module.
- Shallow Copy: It is a bitwise copy of an object. The created copy object has the exact copy of values in the original object. It copies the values of references to objects or just the reference address for the same is copied.
- Deep Copy: It copies all values recursively from source to target object and even duplicates the objects referred by the source objects.
Example:
from copy import copy, deepcopy
list_1 = [1, 2, [3, 5], 4]
## shallow copy
list_2 = copy(list_1)
list_2[3] = 9
list_2[2].append(7)
list_2 # output => [1, 2, [3, 5, 7], 9]
list_1 # output => [1, 2, [3, 5, 7], 4]
## deep copy
list_3 = deepcopy(list_1)
list_3[3] = 11
list_3[2].append(9)
list_3 # output => [1, 2, [3, 5, 7, 9], 11]
list_1 # output => [1, 2, [3, 5, 7], 4]
What is ”__init__”?
Ans: The “__init__” is a constructor method in python which automatically allocates memory when a new object/instance is created. All the classes in python have “__init__ “method associated with them. It distinguishes the methods and attributes of a class in local variables.
Example:
# class definition
class Student:
def __init__(self, fname, lname, age, section):
self.firstname = fname
self.lastname = lname
self.age = age
self.section = section
# creating a new object
stu1 = Student("James", "Nicholas", 18, "A1")
What is the difference between python arrays and lists?
Ans:
Arrays:
- Python arrays can contain the same data types of elements.
- The data type of an array should be homogenous.
- It consumes very less memory compared to lists as it is a thin wrapper around arrays of C language.
Lists:
- Python lists can contain different data type of elements.
- The data type of a list can be heterogeneous.
- It costumes large memory which is a disadvantage.
What is the difference between lists and tuples in python?
Ans:
Lists:
- The lists are mutable which can be edited.
- Python lists are processed slowly than tuples.
- Lists are enclosed inside square brackets.
Syntax: list_1 = [3, ‘Thomas’, 7]
Tuples:
- The tuples are immutable which can’t be edited.
- Python tuples are processed fastly than lists.
- Tuples are enclosed inside normal brackets.
Syntax: tup_1 = (3, Thomas, 7)
How are classes created in python?
Ans: Python classes are created using the keyword “class”.
Example:
class Candidate:
def __init__(self, name):
self.name = name
C1=Candidate("xyz")
print(C1.name)
Output: xyz
Explain Inheritance in python.
Ans: Inheritance allows a class to acquire the properties of another class. Inheritance provides the code reusability which makes easier to create and maintain an application. The class from which it is inheriting the properties is called a super class/base/parent class and the class that is inherited is called derived/child class. The different types of inheritance in python are.
- Single inheritance - A derived class acquires the members of a single super class.
- Multi-level inheritance - Assume A as the main base class and B, C are the derived sub-classes for A. If class C is a derived class which is inherited from its class B then this class B becomes base class for C and if this class B is inherited from its base class A then this class A becomes the base class for class B. This level of inheritance is called as multi-level inheritance.
IMAGE
- Hierarchical inheritance - If from one base class you can inherit any number of child classes than it is known as hierarchical inheritance.
- Multiple inheritance - If a derived class is inherited from more than one base class then it is known as multiple inheritance.
What is lambda in Python? Why is it used?
Ans: Lambda in python is an anonymous function that accepts any number of arguments but can have a single expression. It is used in situations which requires the anonymous function for short during of time and is used in two ways.
- Assigning lambda functions to a variable.
Example:
mul = lambda a, b : a * b
print(mul(3, 7)) # output => 21
2.Wrapping lambda functions inside another function.
Example:
def myWrapper(n):
return lambda a : a * n
mulFive = myWrapper(3)
print(mulFive(5)) # output => 125
. What is Pass in Python?
Ans: The keyword “pass” represents a null operation in python. In general, it is used in filling the empty blocks of code which may execute during the run time but has yet to be written.
Example:
def myFunc():
# do nothing
pass
myFunc() # nothing happens
. How is memory managed in Python?
Ans:
- Memory management in python is managed by “Python private heap space”. All the data structures and objects are located in a private heap. Only the python interpreter will take care of the private heap and no programmer can access it.
- The heap space allocation for Python objects is done by python’s memory manager. The core API gives access to certain tools by which the programmers can code.
- Python also contains the inbuild garbage collector which recycles all unused memory and makes it available to heap space.
. What is docstring in Python?
Ans:
- docstring or a documentation string is a multiple-line string which documents a specific code segment.
- The docstring describes what a function or method does.
- These docstrings are specified within triple quotes. It cannot be assigned to any variable and serves the purpose of comments as well.
Example:
"""
Using docstring as a comment.
This code divides 2 numbers
"""
x=10
y=5
z=x/y
print(z)
Output: 2.0
. How are arguments passed by value or by reference in python?
Ans:
Pass by value: It means that the copy of an actual object is passed such that changing the copy of an object will not change the value of an original object.
Pass by reference: It means that the reference to an object is passed such that changing the new object will change the value of an original object.
In Python, arguments are passed only by reference.
Example:
def affixNumber(arr):
arr.append(5)
arr = [1, 2, 3, 4]
print(arr) #Output: => [1, 2, 3, 4]
affixNumber(arr)
print(arr) #Output: => [1, 2, 3, 4, 5]
. What is the purpose of is, not and in operators?
Ans: The operators are the special functions which take one or more values for producing the corresponding results.
is: The “is” operator returns true when the two operands are true.
not: The “not” operator returns the inverse of a boolean value.
in: The “in” operator will check if some element is present in some sequence.
. What is a dictionary in Python?
Ans: Dictionary is the built-in data types in Python. The dictionaries define the one-to-one relationship between keys and values. It contains the pair of keys with its corresponding values and is indexed by keys.
Example:
dict={'State':'Telangana','District':'Hyderabad','Locality':'Charminar'}
|
1
|
print dict[State]
|
Telangana
1
|
print dict[District]
|
Hyderabad
1
|
print dict[Locality]
|
Charminar
In this example, the dictionary contains the key elements as State, District, Locality and their corresponding values as Telangana, Hyderabad and Charminar respectively.
. Name a few python libraries.
Ans: Python libraries are a collection of python packages. The most frequently used python libraries are.
- Numpy
- Pandas
- Matplotlib
- Scikit-learn
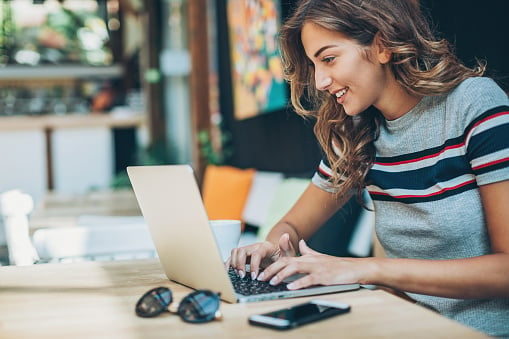
Python Training Certification
- Master Your Craft
- Lifetime LMS & Faculty Access
- 24/7 online expert support
- Real-world & Project Based Learning
. Explain split(), sub(), subn() methods of “re” module in python.
Ans: The “re” module in python provides these three methods for modifying the strings.
- split() - This method uses the regex pattern for splitting a given string into a list.
- sub() - This method finds all the substrings for the matched regex pattern and then replaces it with a different string.
- subn() - It is similar to sub() method and returns a new string along with number of replacements.
. How is python numpy better than lists?
Ans: Python NumPy is used instead of lists because of the following reasons.
- Consumes less memory.
- Performs fast.
- Very convenient.
. What is the difference between NumPy and SciPy?
Ans:
- NumPy contains the array data types and basic operations that include indexing, sorting, reshaping, basic functions, et cetera while SciPy contains all the numeric code.
- Both NumPy and SciPy contains the linear algebra functions. NumPy contains only fewer functions but SciPy contains the full-featured version of linear algebra modules as well as other numerical algorithms.
- For scientific computing, both NumPy and SciPy will be installed but SciPy provides more features compared to NumPy.
. How do you make 3D plots/visualizations using NumPy/SciPy?
Ans: As 2D plotting, 3D graphics is beyond the scope of NumPy and SciPy but there are certain packages in python that integrate with NumPy. The Matplotlib library provides basic 3D plotting in “mplot3d” subpackage. The “MayaVi” provides a wide range of high-quality 3D visualization features which utilizes the power of VTK engine.
. What is the process of compilation and linking in Python?
Ans: The process of compilation and linking allows the new extensions for proper compilation without any errors and linking is done as it passes the compiled procedure. The python interpreter is used for providing the dynamic loading to set up the configuration files and rebuild the interpreter. The steps of process include.
- Create a file of any language supported by the compiler. Eg. file.c or file.cpp
- Place the file in the directory/module of distribution which will be used.
- Add a line in the file Setup.local that is present in modules/directory.
- Run the file using spam file.o
- Once it runs successfully then rebuild the interpreter by using make command on the top-level directory.
- If the file changes then run “rebuildMakefile” by using the command “make Makefile”.
. How does break, continue and pass work in Python?
Ans:
Break: It terminates the loop after the condition is met and then the control will be transferred to the next statement.
Continue: It skips some part of a loop when the condition is met and then the control will be transferred to the beginning of the loop.
Pass: It is used when you need some block of code syntactically but must skip its execution. It is basically a null operation and nothing happens when executed.
. What is pickling and unpickling?
Ans:
Pickling is a process of a python module which accepts a python object and converts it into a string representation and then dumps it into a file by using a dump function.
Unpickling is a process of retrieving the original python objects from the stored string representation.
. How to comment multiple lines in Python?
Ans: The commenting of a line is generally prefixed by a “#” character. The multi-line comments must be specified by prefixing with “#” character for each line. To do this, hold the “ctrl” key and “left-click” with mouse button in every place where you need a “#” character in that line.
. How do you add the values to a python array?
Ans: The elements are added to an array by using append(), extend() and insert(i,x) functions.
Example:
a=arr.array('d', [1.5 , 2.5 ,3.5] )
a.append(5.6)
print(a)
a.extend([2.3,4.1,6.5])
print(a)
a.insert(3,1.8)
print(a)
Output:
array(‘d’, [1.5, 2.5, 3.5, 5.6])
array(‘d’, [1.5, 2.5, 3.5, 5.6, 2.3, 4.1, 6.5])
array(‘d’, [1.5, 2.5, 3.5, 1.8, 5.6, 2.3, 4.1, 6.5])
. What is the difference between .py and .pyc files?
Ans:
- The .py files contain the source code of a program while .pyc files contain the byte code of a program. The byte code is generated after the compilation of .py file. The .pyc files are only created for the file that you import but are not created for all files which you run.
- The python interpreter checks for the compiled files before a program is executed. If the file is present than virtual machine executes it, if not found then it checks for .py file and if it is found then it compiles it to .pyc file and VM executes it.
- The compilation time is saved when you have a .pyc file.
Subscribe to our YouTube channel to get new updates..!
. What is slicing in python?
Ans: As the name suggests the slicing is to take the parts of a list or tuple.
Syntax: [start : stop : step]
start: It represents the starting index from where to slice a list or tuple.
stop: It represents the ending index where it should stop.
step: It represents the number of steps required to jump.
The default values for start is 0, step is 1 and stop is the number of items in it.
Slicing is applicable on strings, arrays, lists and tuples.
[ Related Article: slicing in python ]
Example:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
print(numbers[1 : : 2])
output : [2, 4, 6, 8, 10]
. What does *args and **kwargs mean?
Ans:
*args:
- The *args is a special syntax used in a function definition for passing a variable-length argument.
- “*” indicates a variable length and “args” is the name used by convention. It can be used by any other.
Example:
def multiply(x, y, *argv):
mul = x * y
for num in argv:
mul *= num
return mul
print(multiply(1, 2, 3, 4, 5))
Output: 120
**kwargs:
- The **kwargs is a special syntax used in a function definition for passing a variable-length keyworded argument.
- Here **kwargs used by convention. It can be used by any other name.
- Keyworded argument is a variable that has a name when it’s passed to a function.
- It is actually a dictionary of the variable name and its value.
Example:
def tellArguments(**kwargs):
for key, value in kwargs.items():
print(key + ": " + value)
tellArguments(arg1 = "argument 1", arg2 = "argument 2", arg3 = "argument 3")
Output:
arg1: argument 1
arg2: argument 2
arg3: argument 3
. What are negative indexes and why are they used?
Ans:
- The negative indexes are the indexes from the end of the list or tuple or string.
- If the index is specified with a negative number such that Arr[-1], then it means the last element of array Arr[].
Example:
arr = [1, 3, 5, 7, 9, 11]
To get the last element, use the below statement.
print(arr[-1])
Output: 11
To get the second last element then use the below statement.
print(arr[-2])
Output: 9
. Mention the differences between Django, Pyramid and Flask libraries.
Ans:
- Flask is a “microframework“ which is built primarily with simple requirements for running the small applications. Flask is ready to use the external libraries.
- The pyramid is preferred to build large applications which provide flexibility to the developers in using the right tools for their project. The pyramid is configured heavily and lets the developer choose the database, URL structure, templating style and more.
- Django includes an ORM and can also be used for large applications just like Pyramid.
. What Is Python?
Ans: Python is an interpreter, interactive, object-oriented programming language. It incorporates modules, exceptions, dynamic typing, very high level dynamic data types, and classes. Python combines remarkable power with very clear syntax. It has interfaces to many system calls and libraries, as well as to various window systems, and is extensible in C or C++. It is also usable as an extension language for applications that need a programmable interface. Finally, Python is portable: it runs on many Unix variants, on the Mac, and on PCs under MS-DOS, Windows, Windows NT, and OS/2.
. How Can I Find The Methods Or Attributes Of An Object?
Ans: For an instance x of a user-defined class, dir(x) returns an alphabetized list of the names containing the instance attributes and methods and attributes defined by its class.
. What Is A Class?
Ans:
- A class is the particular object type created by executing a class statement. Class objects are used as templates to create instance objects, which embody both the data (attributes) and code (methods) specific to a data type.
- A class can be based on one or more other classes, called its base class (es). It then inherits the attributes and methods of its base classes. This allows an object model to be successively refined by inheritance. You might have a generic Mailbox class that provides basic accessory methods for a mailbox, and subclasses such as MboxMailbox, MaildirMailbox, Outlook Mailbox that handle various specific mailbox formats.
. What Is Self?
Ans: Self is merely a conventional name for the first argument of a method. A method defined as meth(self, a, b, c) should be called as x.meth(a, b, c) for some instance x of the class in which the definition occurs; the called method will think it is called as meth(x, a, b, c).
If you have any doubts on python , then get them clarified from python Industry experts on our Python Community !
. How Do I Run A Sub process With Pipes Connected To Both Input And Output?
Ans: Use the popen2 module.
For example:
Import popen2
From child, to child = popen2.popen2("command")
tochild.write ("inputn")
tochild.flush ()
Output = fromchild.readline ()
. Can I Create My Own Functions In C?
Ans: Yes,you can create built-in modules containing functions, variables,exceptions and even new types in C.
. Can I Create My Own Functions In C++?
Ans : Yes,using the C compatibility features found in C++. Place extern "C" { ... } around the Python include files and put extern "C" before each function that is going to be called by the Python interpreter. Global or static C++ objects with constructors are probably not a good idea.
. How Can I Execute Arbitrary Python Statements From C?
Ans: The highest-level function to do this is PyRun_SimpleString () which takes a single string argument to be executed in the context of the module __main__ and returns 0 for success and -1 when an exception occurred (including Syntax Error).
. How Do I Interface To C++ Objects From Python?
Ans: Depending on your requirements, there are many approaches. To do this manually, begin by reading the "Extending and Embedding" document.Realize that for the Python run-time system, there isn't a whole lot of difference between C and C++ -- so the strategy of building a new Python type around a C structure (pointer) type will also work for C++ objects.
. How Do I Make Python Scripts Executable?
Ans:
- On Windows 2000, the standard Python installer already associates the .py extension with a file type (Python. File) and gives that file type an open command that runs the interpreter (D: Program FilesPythonpython.exe "%1" %*). This is enough to make scripts executable from the command prompt as 'foo.py'. If you'd rather be able to execute the script by simple typing 'food' with no extension you need to add .py to the PATHEXT environment variable.
- On Windows NT, the steps taken by the installer as described above allow you to run a script with 'foo.py', but a longtime bug in the NT command processor prevents you from redirecting the input or output of any script executed in this way. This is often important.
- The incantation for making a Python script executable under WinNT is to give the file an extension of .cmd and add the following as the first line:
@setlocal enable extensions & python -x %~f0 %* & go to :EOF
. How Do I Debug An Extension?
Ans : When using GDB with dynamically loaded extensions, you can't set a breakpoint in your extension until your extension is loaded.
In your .gdbinit file (or interactively), add the command:
Br _PyImport_LoadDynamicModule
Then, when you run GDB:
$ Gdb /local/bin/python
Gdb) run myscript.py
Gdb) continue # repeat until your extension is loaded
Gdb) finish # so that your extension is loaded
Gdb) br myfunction.c:50
Gdb) continue
. Where Is Freeze For Windows?
Ans:"Freeze" is a program that allows you to ship a Python program as a single stand-alone executable file. It is not a compiler;your programs don't run any faster, but they are more easily distributable, at least to platforms with the same OS and CPU.
Upcoming Python Training Certification Online classes
Batch starts on 9th Aug 2025 |
|
||
Batch starts on 13th Aug 2025 |
|
||
Batch starts on 17th Aug 2025 |
|