PHP interview questions
Last updated on Nov 17, 2023
PHP is an acronym for PHP Hypertext Preprocessor. It is a widely implemented open-source programming language which is used in creating dynamic websites and mobile API’s.
In this article, you can go through the set of PHP interview questions most frequently asked in the interview panel. This will help you crack the interview as the topmost industry experts curate these at HKR trainings.
Most Frequently Asked PHP Interview Questions
Let us have a quick review of the PHP interview questions.
What is PHP?
Ans: PHP is a server-side scripting language generally used for web applications. PHP has many frameworks and cms for creating websites. Even a non-technical person is able to create the sites using its CMS.WordPress, osCommerce which are the famous CMS of PHP. It is also an object-oriented programming language like java, C-sharp etc. It is very easy for learning.
What are the different types of PHP variables?
Ans: There are eight different data types of PHP used to construct the variables.
Integers: These are the whole numbers, without a decimal point, like 4195.
Doubles: These are the floating-point numbers, like 3.14159 or 49.1.
Booleans: This type will have only two possible values either true or false.
NULL: It is a special type that only has one value: NULL.
Strings: These are sequences of characters, like ‘PHP supports string operations.’
Arrays: These are named and indexed collections of other values.
Objects: These are instances of programmer-defined classes, which can package up both other kinds of values and functions that are specific to the class.
Resources: These are special variables that hold references to resources external to PHP.
What are constructor and destructor in PHP?
Ans: The constructor and destructor are special type functions in PHP which are automatically called when a PHP class object is created and destroyed. The constructor is the most useful among these two because it allows you to send parameters along while creating a new object, which can then be used to initialize variables on the object. The below example of constructor and destructor in PHP.
Example:
}
public function setLink(Foo $link){
$this->;link = $link;
}
public function __destruct() {
echo 'Destroying: ', $this->name, PHP_EOL;
}
}
?>
What does PEAR stands for?
Ans: PEAR means “PHP Extension and Application Repository”. it extends PHP and provides a higher level of programming for web developers
What are the different types of Array in PHP?
Ans: There are three different types of Arrays in PHP.
Indexed Array: An array with a numeric index is known as the indexed array. Here the values are stored and accessed in a linear fashion.
Associative Array: An array with strings as the index is known as the associative array. This stores element values in association with key values rather than in strict linear index order.
Multidimensional Array: An array which contains one or more arrays is known as a multidimensional array. The values are accessed using multiple indices.
What is the difference between the single-quoted string and double-quoted string?
Ans: Singly quoted strings are literally treated, whereas doubly quoted strings replace variables with their values as well as specially interpreting certain character sequences.
Example:
$variable = "name";
$statement = 'This $variable will not print!n';
print($statement);
print "
;"
$statement = "This $variable will print!n"
print($statement);
?>
Output:
My $variable will not print!
My name will print
How to execute an SQL query? How to fetch its result?
Ans:
The below statement is used for executing an SQL query.
$my_qry = mysql_query(“SELECT * FROM `users` WHERE `u_id`=’1′; “);
The below statement is used for fetching the result of SQL query.
$result = mysql_fetch_array($my_qry);
The below statement is used for printing the result of the SQL query.
echo $result[‘First_name’];
What is the difference between “echo” and “print” in PHP?
Ans:
Echo:
- PHP echo displays one or more strings as output.
- PHP echo is not a function, it is a language construct. Hence use of parentheses is not required. But when there is a need to pass more than one parameter to echo, then the use of parentheses is required.
- Echo is faster than print as it doesn’t return any value.
Print:
- PHP print displays a string as output.
- PHP print is also a language construct but not a function. Here the use of parentheses is not required with the argument list. Unlike echo, it always returns 1.
- The print is slow compared to the echo.
How can we display the output directly to the browser?
Ans: To be able to display the output directly to the browser, we have to use the special tags .
. What is the main difference between PHP 4 and PHP 5?
Ans:
. Is multiple inheritances supported in PHP?
Ans:
Is it possible to inherit from multiple parent classes simultaneously in PHP?
No, PHP does not support inheriting from multiple parent classes simultaneously.
Can a class be extended from more than one class in PHP?
No, a class in PHP cannot be extended from more than one class.
What is the limitation of inheritance in PHP?
The limitation of inheritance in PHP is that it only allows for single inheritance.
Does PHP support multiple inheritance?
No, PHP only supports single inheritance.
. What is the meaning of a final class and a final method?
Ans: ‘Final’ is introduced in PHP5. Final class means that this class cannot be extended and a final method cannot be override.
. How comparison of objects is done in PHP5?
Ans: We use the operator ‘==’ to test is two object are instanced from the same class and have same attributes and equal values. We can test if two object are refering to the same instance of the same class by the use of the identity operator ‘===’.
. How can PHP and HTML interact?
Ans: It is possible to generate HTML through PHP scripts, and it is possible to pass information’s from HTML to PHP.
. What is the difference between $var and $$var?
Ans: Both “$var” and “$$var” are variables. The “$var” is a variable with a fixed name while ”$$var” is a variable whose name is stored in “$var”.
Example: If “$var” contains “message” then “$$var” is the same as “$message"
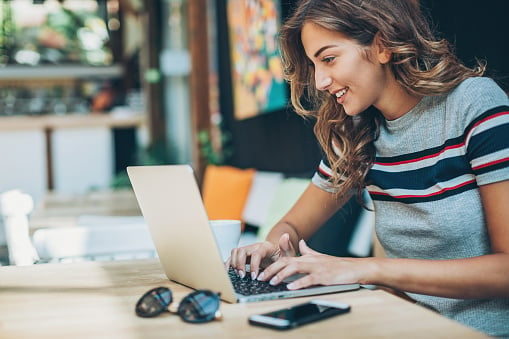
PHP Training Certification
- Master Your Craft
- Lifetime LMS & Faculty Access
- 24/7 online expert support
- Real-world & Project Based Learning
. What is the use of callback in PHP?
Ans:
- PHP callback are the functions which are called dynamically by PHP.
- They are used by native functions such as array_map, usort, preg_replace_callback, etc.
- A callback function is a function that is created and is then passed to another function as an argument. Once it has access to the callback function, the receiving function can then call it whenever there is a need.
Example:
function thisFuncTakesACallback($callbackFunc)
{
echo "I'm going to call $callbackFunc!
";
$callbackFunc();
}
function thisFuncGetsCalled()
{
echo "I'm a callback function!
";
}
thisFuncTakesACallback( 'thisFuncGetsCalled' );
?>
. How can we execute a PHP script using a command line?
Ans: Run the PHP CLI (Command-line Interface) program and provide the PHP script file name as the command-line argument.
Example:
php myScript.php
Assuming php as the command to invoke the CLI program.
Keep in mind that if the PHP script is written for the Web CGI interface, it may not execute properly in the command-line environment.
. How to submit a form without a submit button?
Ans:
A form can be submitted without any button in the following ways.
- On the “OnClick” event of a label in the form, a JavaScript function can be called to submit the form.
Example: document.form_name.submit()
2. Submitting using a Hyperlink. On clicking the link, a JavaScript function can be called.
Example: <a.href="javascript:document.MyForm.submit();">
3. A form can be also be submitted in the following ways without using any submit button:
- Submitting a form by clicking a link.
- Submitting a form by selecting an option from the drop-down box with the invocation of an “onChange” event.
- By using JavaScript: document.form.submit();
4. By using the header(“location:page.php”);
. What library is used for PDF in PHP?
Ans: The PDF files are created with PDF functions by using PDFlib version 6. PDFlib offers an object-oriented API for PHP5 in addition to the function-oriented API for PHP4.
There is also the » Panda module.
FPDF is a PHP class, which allows generating PDF files with pure PHP, without using PDFlib. F from FPDF stands for Free, it can be used for any requirement and modify them to suit the needs. FPDF doesn’t require any extension except zlib to activate compression and GD for GIF support and it works with PHP4 and PHP5.
. How can you encrypt a password using PHP?
Ans: The “crypt()” function is used to create one-way encryption. It takes one input string and one optional parameter. The function is defined as
crypt(input_string, salt)
where “input_string” consists of the string that has to be encrypted and “salt” is an optional parameter.
The PHP uses DES for encryption. The format is as follows:
$password=crypt('hkrtrainings');
print $password."is the encrypted version of hkrtrainings";
?>
Subscribe to our YouTube channel to get new updates..!
. Does PHP support variable-length argument functions?
Ans: Yes, PHP supports the use of variable length argument functions. You are able to pass any number of arguments to a function. The syntax includes using three dots before the argument name as shown in the below example:
function add(...$num) {
$sum = 0;
foreach ($num as $n) {
$sum += $n;
}
return $sum;
}
echo add(5, 6, 7, 8);
?>
Output: 26
. What are the different types of errors in PHP?
Ans: There are three different types of runtime errors in PHP.
1. Notices: These errors are trivial and non-critical that PHP encounters while executing a script.
Example: Accessing a variable which is not yet defined.
By default, such errors are not displayed to the user at all, although you can change this default behaviour.
2. Warnings: These errors are more serious.
Example: Attempting to include() a file which does not exist.
By default, these errors are displayed to the user, but they do not result in script termination.
3. Fatal errors: These errors are very critical.
Example: Instantiating an object of a non-existent class, or calling a non-existent function.
These errors cause the immediate termination of the script, and PHP’s default behaviour is to display them to the user when they take place.
. How are comments used in PHP?
Ans: There are two ways of using the comments in PHP.
- Single line comments that can be used using the conventional ‘#’ sign.
Example:
# This is a comment
echo "Single-line comment";
?>
2. Multi-line comments that can be denoted using ‘/* */’ in PHP.
Example:
/*
This is
a
Multi-line
Comment
In PHP;
*/
echo "Multi-line comment";
?>
. Differentiate between an indexed array and an associative array.
Ans:
Indexed Arrays:
These arrays will have elements that contain a numerical index value.
Example: $color=array("red","green","blue");
Here, red is at index 0, green at index 1, and blue at index 2.
Associative Arrays:
These arrays hold the elements with string indices as shown below:
Example: $salary=array("Jacob"=>"20000","John"=>"44000","Josh"=>"60000");
. How can you get the IP address of a client in PHP?
Ans: It is very easy to get an IP address of a client who is connected by using the following syntax.
$_SERVER["REMOTE_ADDR"];
. How can we increase the execution time of a PHP script?
Ans: The default time allowed for a PHP script for execution is 30 seconds mentioned in the “php.ini” file. The function that will be used is
“set_time_limit(int sec)”.
If the value passed is ‘0’, then it takes unlimited time. Note that if the default timer is set to 30 seconds and 20 seconds is specified in “set_time_limit()”, then the script will run for 45 seconds.
- This time can be increased by altering “max_execution_time” in seconds. The time must be changed accordingly keeping the environment of the server. This is because altering the execution time will also affect all the sites hosted by the server.
- The script execution time can be increased by:
- Using the “sleep()” function in the PHP script.
- Using the “set_time_limit()” function.
The default limit is 30 seconds. The time limit can be set to zero to impose no time limit.
. What are the steps to create a new database using MySQL and PHP?
Ans: There are four steps in creating a new database using MySQL and PHP.
- A connection is established to the MySQL server using the PHP script.
- The connection is then validated. If the connection is successful, then you are allowed to write a sample query for verification.
- Queries that create the database are input and later stored into a string variable.
- Then, the created queries are executed one after the other.
. What is the difference between “mysql_connect” and “mysql_pconnect”?
Ans:
- The mysql_pconnect() method is used to make a persistent connection to the database which means a SQL link that doesn’t close the connection even after the execution of the script ends.
- The mysql_connect() method simply provides only the new database connection while using mysql_pconnect(), the method would first try to find a persistent link that’s already open with the same host, username and password.
- If one is found, an identifier for it will be returned instead of opening a new connection. The connection to the SQL server is not closed when the execution of the script ends rather the link will remain open for future use.
. What is Zend Engine?
Ans:
- Zend Engine is internally used by PHP as a compiler and a runtime engine.
- PHP Scripts are loaded into memory and are compiled into Zend OPCodes.
- These OPCodes are executed and the generated HTML is sent to the client.
- It provides memory and resource management services and other standard services for the PHP language.
- The performance, reliability, and extensibility of the Zend Engine have a significant role.
. What is Type hinting in PHP?
Ans:
- Type hinting is used to specify the expected data type of an argument in a function declaration.
- When a function is called, PHP will then check whether or not the method arguments are of the specified type. If not, the run-time will raise an error and execution will be halted.
Example:
function sendEmail (Email $email)
{
$email->send();
}
?>
The above example describes how to send the Email function argument “$email” Type hinted of an “Email” Class. It means to call this function you must have to pass an email object otherwise an error is generated.
. What is htaccess? Why do we use it and where?
Ans:
- The “.htaccess” files are the configuration files of Apache Server that provide settings for configuration changes on a per-directory basis.
- A file which contains one or more configuration directives is placed into a particular document directory; the directives apply to that directory and all subdirectories thereof.
- The “.htaccess” files are used in changing the functionality and features of the Apache web server.
For instance:
- The “.htaccess” file is used for rewriting the URL.
- It is used for making the site as password-protected.
- It restricts few IP addresses such that those sites cannot be opened on these restricted IP addresses.
. How to create a session? How to set a value in session? How to Remove data from a session?
Ans: The following are the ways of creating, setting and removing a session.
- Creating a session : session_start();
- Setting a value into session : $_SESSION[‘USER_ID’]=1;
- Remove the data from a session : unset($_SESSION[‘USER_ID’]);
. What is the use of session and cookies in PHP?
Ans: A session is a global variable stored on the server. a unique id is assigned for each session which is used to retrieve stored values. Sessions can store large data as compared to cookies which have high storage capabilities. The session values will be deleted automatically when the browser is closed.
Creating a cookie in PHP:
Example:
$cookie_value = "hkrtrainings";
setcookie("hkrtrainings",$cookie_value,time()+3600,"/your_usename/","hkrtrainings.com", 1, 1);
if (isset($_COOKIE['cookie']))
echo $_COOKIE["hkrtrainings"];
?>
Creating a session in PHP:
Example:
session_start();
if( isset( $_SESSION['counter'] ) )
{
$_SESSION['counter'] += 1;
}
else
{
$_SESSION['counter'] = 1;
}
$msg = "You have visited this page". $_SESSION['counter'];
$msg .= "in this session.";
?>
. What are some of the popular frameworks in PHP?
Ans: There are many frameworks in PHP. The most popular frameworks are.
- CodeIgniter
- CakePHP
- Laravel
- Zend
- Phalcon
- Yii 2
. How does the ‘foreach’ loop work in PHP? Give an example.
Ans:
- The “foreach” is a looping construct statement which is used in PHP for iterating and looping through the array data type.
- The “foreach” statement works with every single pass of the value, elements get assigned a value and pointers are incremented. This process is repeated until the end of the array.
Syntax:
foreach(array)
{
Code inside the loop;
}
Example:
$colors = array("red", "blue", "green");
foreach ($colors as $value) {
echo "$value";
}
?>
. What is the difference between require() and require_once() functions?
Ans:
require():
- This method includes a specific file and evaluates that file.
- It is preferred for files with fewer functions.
require_once():
- This method includes the files only if they are not included before.
- It is preferred for files when there many functions.
. How can you open a file in PHP?
Ans:
- PHP supports file operations by providing users with excessive file-related functions.
- To open a file, the “fopen()” method is used. This function can open a file or a URL as per necessity. It takes two arguments: “$filename” and “$mode”.
Syntax: resource fopen(string $filename,string $mode[,bool $use_include_path = false [, resource $context ]] )
. How the result set of Mysql be handled in PHP?
Ans:
When handling the result set of Mysql in PHP, there are several functions available. One such function is mysql_fetch_array, which can be used to retrieve a row from the result set as an array with both numeric and associative keys. Another function is mysql_fetch_assoc, which returns a row as an associative array. Additionally, there is mysql_fetch_object, which retrieves a row as an object, and mysql_fetch_row, which returns a row as a numeric array.
These functions provide developers with different options for accessing and manipulating the result set in PHP. By utilizing these functions, you can easily navigate through each row of the result set and retrieve the desired information. It is important to note, however, that the mysql extension used in these functions has been deprecated as of PHP version 5.5.0. Therefore, it is recommended to use the mysqli extension and its respective functions, such as mysqli_fetch_array, mysqli_fetch_assoc, mysqli_fetch_object, or mysqli_fetch_row, for handling the result set in a more up-to-date and secure manner.<
. What is the function mysql_pconnect() usefull for?
Ans : mysql_pconnect() ensure a persistent connection to the database, it means that the connection do not close when the the PHP script ends.
. What is the function file_get_contents() usefull for?
Ans: file_get_contents() lets reading a file and storing it in a string variable.
Explore PHP Sample Resumes Download & Edit, Get Noticed by Top Employers!
. What should we do to be able to export data into an Excel file?
Ans: The most common and used way is to get data into a format supported by Excel. For example, it is possible to write a .csv file, to choose for example comma as separator between fields and then to open the file with Excel.
. How is it possible to set an infinite execution time for PHP script?
Ans: The set_time_limit(0) added at the beginning of a script sets to infinite the time of execution to not have the PHP error ‘maximum execution time exceeded’. It is also possible to specify this in the php.ini file.
. What is the main difference between require () and require once()?
Ans: Require () and require once () perform the same task except that the second function checks if the PHP script is already included or not before executing it.
(same for include once() and include())
. How failures in execution are handled with include () and require () functions?
Ans: If the function require () cannot access to the file then it ends with a fatal error. However, the include () function gives a warning and the PHP script continues to execute.
. How can PHP and JavaScript interact?
Ans:
How can variables be passed back to PHP from JavaScript?
Variables can be passed back to PHP from JavaScript by including them in the URL parameters. JavaScript can add specific variables to the URL, and PHP can retrieve these variables on the server-side.
What are the limitations of PHP and JavaScript interaction?
The main limitation of PHP and JavaScript interaction is their inability to directly interact due to their different nature, server-side (PHP) and client-side (JavaScript). However, they can overcome this limitation by using methods like generating JavaScript code and passing variables through the URL.
How can PHP and JavaScript interact?
PHP and JavaScript cannot directly interact since PHP is a server-side language and JavaScript is a client-side language. However, they can exchange variables by generating JavaScript code with PHP and passing variables back to PHP via the URL.
What is the difference between characters \034 and \x34?
The difference between characters \034 and \x34 lies in their representation. \034 is octal 34, which means it is a character represented in octal notation. On the other hand, \x34 is hex 34, indicating that it is a character represented in hexadecimal notation. While both notations represent the same value, which is the character with ASCII code 52 in decimal, they are represented differently based on the numbering system used.
What is the difference between ereg_replace() and eregi_replace()?
The main difference between ereg_replace() and eregi_replace() lies in their treatment of case sensitivity when matching alphabetic characters. While both functions are used to replace text in a string, ereg_replace() takes case distinction into account when matching characters, meaning it will only match characters with the exact same case. On the other hand, eregi_replace() ignores case distinction, allowing it to match alphabetic characters regardless of their case. So, if you need a replacement function that considers case sensitivity, you would use ereg_replace(), whereas if you want one that ignores case sensitivity, you would opt for eregi_replace().
What is the difference between the functions strstr() and stristr()?
The functions strstr() and stristr() in programming are used to search for a specific substring within a given string. The main difference between these two functions lies in their case sensitivity.
The strstr() function is case-sensitive, which means it considers uppercase and lowercase letters as distinct characters. When using strstr(), it will return the part of the input string from the first occurrence of the specified substring until the end of the string, considering the exact case of the substring.
On the other hand, the stristr() function is case insensitive. It ignores the distinction between uppercase and lowercase letters when searching for the substring in the input string. If stristr() finds a match, it will return the part of the input string from the first occurrence of the substring until the end of the string, regardless of the case of the substring.
In summary, the main distinction between strstr() and stristr() is that strstr() is case-sensitive, while stristr() is case-insensitive.
How is it possible to parse a configuration file?
To parse a configuration file, one can utilize various methods and techniques. One common approach is to use a scripting language such as PHP. In PHP, there are several functions and techniques available to achieve this.
One approach is to use PHP's built-in functions, such as `file_get_contents`, which allows the contents of the configuration file to be read as a string. Once the contents are obtained, they can be manipulated using string processing functions. For example, using `explode`, the string can be split into an array based on a delimiter, such as a line break or a specific character.
Another powerful method is to utilize PHP's `parse_ini_file` function. This function allows a configuration file to be read and interpreted as an array of key-value pairs. The configuration file must follow the INI file format, which consists of sections, properties, and values. This method provides a convenient and standardized way to access configuration settings.
In some cases, more complex configuration file formats may be used, such as XML or JSON. To handle these formats, PHP provides functions like `simplexml_load_file` for parsing XML files and `json_decode` for parsing JSON files. These functions allow for structured access to the configuration settings contained within these files.
Overall, parsing a configuration file in PHP can be achieved using various methods and functions based on the specific format and requirements of the file. By utilizing the appropriate functions and techniques, the configuration settings can be efficiently extracted and used within the PHP application.
What does the expression Exception::__toString mean?
The expression `Exception::__toString` refers to a method within the Exception class in programming languages like PHP. This method is responsible for providing a string representation of the exception object.
When an exception is thrown in a program, it typically contains relevant information about the error or unexpected condition that occurred. This information is often useful for debugging or logging purposes. However, the default behavior of displaying the exception object itself may not be user-friendly or suitable for various scenarios.
By implementing the `__toString` method in the Exception class, programmers can define a custom string representation for the exception. This allows them to control how the exception is displayed when it is converted to a string, making it more meaningful and informative.
For example, if a custom exception class named `MyException` extends the Exception class, the `__toString` method can be overridden to format the exception message, stack trace, and any other relevant details into a comprehensible string format. This can be particularly helpful when logging or displaying exception information in a user interface.
In summary, the expression `Exception::__toString` represents a method that provides a string representation of an exception object. It allows programmers to define a custom format for displaying exception information, enhancing the readability and usefulness of error messages in their programs.
What is the difference between Exception::getMessage and Exception::getLine?
Exception::getMessage is a method in PHP that allows us to retrieve the message associated with an exception. When an exception is thrown, it often includes a specific message that provides detailed information about the error or problem that occurred. Using the Exception::getMessage method, we can obtain this message and use it for error handling, logging, or displaying to the user.
On the other hand, Exception::getLine is another method in PHP that is used to obtain the line number where the exception was thrown. This can be particularly useful when debugging or investigating issues in code. By retrieving the line number using Exception::getLine, we can quickly identify the specific line in our code that caused the exception.
In summary, Exception::getMessage retrieves the message associated with an exception, while Exception::getLine retrieves the line number where the exception occurred. Both methods serve different purposes but are essential when working with exceptions in PHP for effective error handling and debugging.
What is the goto statement useful for?
The goto statement in PHP is a powerful tool that allows programmers to redirect the flow of execution within their program. Its primary purpose is to enable jumping between different sections of code using target labels. By specifying a label followed by a colon, and then using the goto statement followed by the desired target label, developers can easily navigate through various parts of their code without having to write complex conditional statements or loops.
The goto statement is particularly useful in situations where a specific condition needs to be met in order to proceed with the execution. Instead of nesting conditional statements and complicating the code, a developer can simply use the goto statement to jump directly to the desired section of code when the condition is met.
Furthermore, the goto statement can also be used to handle errors and exceptions in a more concise and readable manner. When an exception occurs or an error is encountered, the goto statement can be employed to redirect the program flow to an error-handling section, allowing for efficient and centralized error management.
Overall, the goto statement provides programmers with a straightforward and efficient way to control the flow of their PHP programs. By utilizing target labels and the goto statement, developers can easily navigate through different sections of their code, handle errors, and improve the readability and maintainability of their programs.
How can we determine whether a PHP variable is an instantiated object of a certain class?
To determine whether a PHP variable is an instantiated object of a certain class, we can make use of the "instanceof" keyword. By using this keyword, we can verify if the variable is an instance of a specific class or one of its child classes.
Here is an example of how we can utilize "instanceof" to check if a PHP variable is an instantiated object of a certain class:
if ($variable instanceof ClassName) {
// The variable is an instantiated object of the ClassName class
} else {
// The variable is either not an object or not an instance of the ClassName class
}
In the above example, replace `ClassName` with the desired class name. If the variable is indeed an instantiated object of that class, the condition within the `if` statement will evaluate to true, allowing you to execute the appropriate logic. Otherwise, if the variable is not an object or not an instance of the specified class, the logic within the `else` block will be executed.
What is the difference between $a != $b and $a !== $b?
The difference between the operators $a != $b and $a !== $b lies in their evaluations. The != operator checks for inequality, meaning it will return TRUE if $a is not equal to $b. On the other hand, the !== operator checks for non-identity. This means it will return TRUE if $a is not only unequal to $b, but also not identical to it. In simple terms, while the != operator focuses solely on inequality, the !== operator looks for both inequality and non-identity between $a and $b.
What does the array operator
The array operator "===" in PHP is used for comparing two arrays. It checks if the two arrays have the same key/value pairs in the same order and of the same types. If all the elements in both arrays are the same and they have the same order, the comparison returns true. However, if the arrays have even a single difference in the key/value pairs or the order of elements, the comparison will return false.
What are the two main string operators?
There are two main string operators in this excerpt.
The first one is the concatenation operator (?.?), which combines two strings by joining them together. It takes two arguments, the left argument and the right argument, and returns their concatenation.
The second operator is (?.=?). This operator is used to append the right argument to the left argument. It takes the left argument, which is a string, and appends the right argument, which is also a string, to it. The result is a new string that includes both the original left argument and the appended right argument.
What is the difference between the
The "BITWISE AND" operator and the "LOGICAL AND" operator are both operators used in programming languages, but they serve different purposes.
The "LOGICAL AND" operator is primarily used for evaluating conditions. When used between two boolean expressions, it returns true only if both of the expressions are true. In other words, it performs a logical comparison and returns a boolean result based on the truth values of the expressions being compared.
On the other hand, the "BITWISE AND" operator is used to perform bitwise operations on binary representations of numbers or variables. When used with two integers, the "BITWISE AND" operator compares the binary representations of the two numbers bit by bit and returns a new number with each bit set to 1 only if the corresponding bits in both numbers are 1. In simpler terms, it performs a bitwise comparison and returns the result by setting the bits that are common in both numbers.
To summarize, the main difference between the "LOGICAL AND" operator and the "BITWISE AND" operator lies in their purpose and the type of operands they work on. The "LOGICAL AND" operator is used for logical comparisons and returns a boolean result, while the "BITWISE AND" operator is used for performing bitwise comparisons and returns a new number with bits set based on the common bits in the operands.
What does the scope of variables mean?
The scope of a variable refers to the specific context or location in a program where the variable is defined and can be accessed. It determines where the variable can be used and accessed within the code. In most cases, PHP variables have a single scope, meaning they are only available within the block of code where they are declared.
For example, if a variable is defined within a function, its scope is limited to that function. It cannot be accessed from outside the function, such as in another function or the main program. This is a way to encapsulate variables and ensure they are not modified or accessed unintentionally by other parts of the code.
Additionally, the scope of a variable also extends to included or required files. This means that if a variable is defined in one file and that file is included or required in another file, the variable can still be accessed in the second file.
Understanding the scope of variables is crucial for writing clean and efficient code. By properly scoping variables, we can control their visibility and prevent conflicts or unintentional modifications. It allows for better organization and separation of code, as different variables can have the same name within different scopes without causing conflicts.
Overall, the scope of variables in PHP defines the access and usage limitations of a variable within a program, ensuring that variables are used appropriately and avoid unintended interactions with other parts of the code.
What does $_ENV mean?
$_ENV is a special predefined variable in PHP that represents an associative array of variables passed to the current PHP script through the environment method. It serves as a way to access and manipulate the environment variables sent to the server when the script is executed. These variables can include information like server paths, user credentials, or custom variables set by the server administrator. By utilizing $_ENV, developers have the ability to access and utilize this environment information within their PHP scripts, allowing for more advanced and dynamic functionality.
How can we get the error when there is a problem uploading a file?
To obtain the error when encountering an issue during file upload, we can access the error code by referencing the superglobal variable $_FILES['userfile']['error']. This variable holds the error code related to the uploaded file. By checking the value of this variable, we can determine the specific error that occurred during the upload process.
What does $_SERVER mean?
$_SERVER is a built-in global variable in PHP that represents an associative array containing information generated by the web server. This array consists of various key-value pairs that provide important details related to the current web server environment. Some of the commonly included information within $_SERVER includes paths, headers, and script locations.
By accessing $_SERVER, developers can easily extract relevant information about the request, such as the user's IP address, user agent, request method, and protocol. Additionally, this array can provide insights into the server's file paths, including the document root, current script file path, and the server's software and version.
In summary, $_SERVER is a versatile variable in PHP that captures crucial information about the web server environment, offering developers a convenient way to access details about the incoming request and the server configuration.
What does $GLOBALS mean?
$GLOBALS is a special associative array in PHP that contains references to all variables currently defined in the global scope of the script. This means that any variable declared outside of a function or class can be accessed using $GLOBALS followed by the variable name.
By using $GLOBALS, you can access and manipulate global variables from anywhere within your script without worrying about scoping issues. It provides a convenient way to simplify the use of global variables and allows for easy modification of their values.
It's important to note that $GLOBALS is always accessible, regardless of the scope within your script. This makes it a powerful tool for managing global state and sharing data throughout your program. However, it's generally recommended to minimize the use of global variables for better code organization and maintainability.
What is the difference between session_unregister() and session_unset()?
session_unregister() and session_unset() are both PHP functions used to manage session variables. However, there are important differences between the two.
The session_unregister() function is used to unregister a specific global variable from the current session. When a variable is unregistered, it is removed from the session and can no longer be accessed or used within that session. This function is particularly useful when you want to remove a specific variable from the session without affecting any other variables that may exist.
On the other hand, session_unset() serves a different purpose. This function is used to free all session variables within the current session. When session_unset() is called, it clears out all session variables, removing all data associated with the session. This is different from session_unregister() as it does not allow you to selectively remove only certain variables, but instead removes all of them.
In summary, session_unregister() unregisters a specific global variable from the current session, while session_unset() clears out all session variables, effectively freeing all the data associated with the session.
When do sessions end?
Sessions in PHP automatically end when the execution of the PHP script is completed. This means that when the script finishes executing all its instructions and tasks, the session will naturally come to an end. However, it is worth noting that sessions can also be manually terminated using the function "session_write_close()". By invoking this function, sessions can be explicitly closed before the script finishes its execution, providing a way to control the end of a session if needed.
What is the meaning of a Persistent Cookie?
A persistent cookie refers to a type of cookie that remains stored on the browser's computer for an extended period of time. Unlike temporary cookies, which are deleted once the browser is closed, persistent cookies are permanently saved in a cookie file. These cookies are designed to retain information about user preferences, settings, or login credentials so that when the user revisits a website, their previous preferences can be remembered and applied. This allows for a more personalized browsing experience and eliminates the need for users to repeatedly enter their information.
In PHP, objects are passed by value or by reference?
In PHP, objects are passed by value or by reference depending on the specific scenario. By default, objects are passed by reference, which means that when an object is assigned to a variable or passed as an argument to a function, the variable or parameter holds a reference to the original object. This means that any changes made to the object within the function or through the variable will affect the original object outside of the function. However, in certain cases, objects can be passed by value. This happens when using the assignment operator (=) to assign one object variable to another, or when passing an object as an argument to a function or method that explicitly specifies passing by value using the "&" operator. In these situations, a copy of the object is made, and any changes made to the object within the function or through the variable will not affect the original object outside of the function. It is important to note that passing objects by reference or by value also depends on the PHP version being used. Prior to PHP 5, objects were always passed by value, but starting from PHP 5, they are passed by reference by default.
If the variable $var1 is set to 10 and the $var2 is set to the character var1, what?s the value of $$var2?
If the variable $var1 is set to 10 and the $var2 is set to the character var1, the value of $$var2 would be the value of the variable with the name stored in $var2, which in this case is 'var1'. Therefore, $$var2 would be equal to the value of the variable $var1, which is 10.
When is a conditional statement ended with endif?
A conditional statement is ended with "endif" when the original "if" statement does not have braces and is followed directly by the code block. In other words, if the "if" statement is not enclosed in curly braces and is immediately followed by the code to be executed, the conditional statement is terminated with "endif".
How is it possible to cast types in PHP?
In PHP, casting types allows you to convert a value from one data type to another. You can accomplish this by specifying the desired output type within parentheses before the variable you want to cast. Here are the different casting options available in PHP:
- To convert a value to an integer, you can use either `(int)` or `(integer)`.
- For boolean conversion, you can use either `(bool)` or `(boolean)`.
- To transform a value into a floating-point number, you can use `(float)`, `(double)`, or `(real)`.
- If you need to convert a value into a string, you can use `(string)`.
- To cast a value into an array, use `(array)`.
- Finally, if you want to convert a value into an object, you can use `(object)`.
By utilizing these casting techniques, you can easily change the data type of a variable in PHP to suit your specific needs.
Will a comparison of an integer 12 and a string ?13? work in PHP?
In PHP, a comparison can be made between an integer and a string using certain rules. However, it is important to note that PHP will automatically cast the string to an integer for the purpose of the comparison.
In the given scenario, if we compare the integer 12 with the string "?13?", PHP will cast the string to an integer, resulting in the value 13. Thus, the comparison will be between the integers 12 and 13.
In PHP, when comparing two integers, the comparison will evaluate to true if the first integer is less than the second integer. Otherwise, it will evaluate to false.
So, if you compare the integer 12 and the string "?13?" in PHP, the comparison will evaluate to false since 12 is not less than 13.
How is a constant defined in a PHP script?
In a PHP script, a constant can be defined using the `define()` directive. This directive allows us to assign a specific value to a constant. To define a constant, we use the `define()` function followed by the constant name, and the value we want to assign to it. For example:
```php
define('ACONSTANT', 123);
```
In this example, we have defined a constant named "ACONSTANT" with a value of 123. Once defined, the value of a constant cannot be changed throughout the execution of the PHP script.
What is the most convenient hashing method to be used to hash passwords?
When it comes to selecting a hashing method for password encryption, it is recommended to avoid using common algorithms such as md5, sha1, or sha256. While these algorithms are designed to be fast, they can introduce vulnerabilities when used for hashing passwords.
Instead, it is more preferable to use the crypt() function, as it inherently supports multiple hashing algorithms. This provides flexibility and allows for greater customization based on specific security requirements. Additionally, the hash() function is another viable option as it supports an even wider range of variants compared to crypt().
By opting for crypt() or hash(), which offer greater versatility and advanced encryption options, the security of password hashing can be significantly enhanced. It is important to prioritize security over convenience to ensure the protection of user passwords.
How is it possible to return a value from a function?
Returning a value from a function is made possible through the use of the 'return' statement followed by the desired value. When executing a function, if a specific value needs to be passed back to the part of the program that called it, the 'return' statement is used to achieve this. By including the keyword 'return' followed by the value to be returned, the function will terminate and the specified value will be sent back to the caller. This allows for the seamless flow of information between different parts of a program, enabling the utilization of function results for further processing or output.
How can we define a variable accessible in functions of a PHP script?
To define a variable that is accessible in functions of a PHP script, the global keyword can be used. This feature allows us to specify that a variable is global, meaning it can be accessed and modified from anywhere within the PHP script, including inside functions.
By declaring a variable as global within a function, we are essentially instructing PHP to make that variable available outside of the function's scope. This way, the variable can be accessed, modified, or used in other functions or parts of the script.
To make a variable global, we need to use the global keyword followed by the variable name inside the function. This informs PHP that we want to use the variable from the global scope rather than creating a new local variable with the same name.
Here's an example of how to define a global variable in PHP:
```
$globalVariable = "This is a global variable";
function myFunction() {
global $globalVariable;
// Now we can use $globalVariable inside this function
echo $globalVariable;
}
myFunction(); // Output: This is a global variable
```
In the example above, we declare the variable `$globalVariable` outside of the function and then use the global keyword inside the function to make it accessible. This allows us to echo the value of `$globalVariable` from within `myFunction()`.
Using the global keyword enables us to create and manipulate variables that can be accessed by multiple functions within a PHP script, providing a way to share data across different parts of the code. However, it is important to use global variables judiciously, as excessive reliance on them can make code harder to understand and maintain.
Is it possible to remove the HTML tags from data?
Yes, it is possible to remove HTML tags from data. A commonly used function for this purpose is strip_tags(). By using the strip_tags() function, you can clean a string and eliminate all HTML tags within it. This function effectively filters out any HTML markup, leaving behind only the raw, plain text content. So, if you have a string that contains HTML tags, utilizing the strip_tags() function can help you remove those tags and obtain the clean text data.
How can we automatically escape incoming data?
To automatically escape incoming data in PHP, there are several methods you can employ. One commonly used approach is by utilizing PHP's built-in functions for data sanitization and validation.
Firstly, you can utilize the htmlentities() function to convert special characters in the incoming data to their corresponding HTML entities. This helps prevent any potentially malicious code from being executed when the data is displayed.
Additionally, you can use the addslashes() function to add backslashes before characters like single quotes, double quotes, and backslashes themselves. This prevents any unintended interpretation of these characters and ensures the data is treated as a literal string.
Another effective method is to utilize prepared statements with parameterized queries when interacting with databases. This approach separates the query logic from the user-provided data, making it nearly impossible for malicious input to be executed as part of a database query.
Furthermore, considering the usage of input validation is crucial. PHP provides various validation functions, such as filter_var(), which allow you to verify the format and integrity of the incoming data. By enforcing strict validation rules, you can ensure that only legitimate and expected data is processed.
In addition to these techniques, it is essential to keep your PHP installation up to date to benefit from the latest security enhancements and bug fixes. Regularly reviewing and patching any known vulnerabilities in the PHP version you are using is crucial for maintaining data integrity and security.
Overall, a combination of data sanitization, parameterized queries, input validation, and keeping your PHP installation updated are effective ways to automatically escape and protect against any potential security vulnerabilities arising from incoming data.
How is it possible to remove escape characters from a string?
To remove escape characters before apostrophes in a string, the strip slashes function can be utilized. This function allows us to extract the string without the presence of any escape characters. By applying the strip slashes function, the escape characters preceding the apostrophes can be eliminated, ensuring a clean string devoid of any unwanted characters.
How do I escape data before storing it in the database?
Answer:
To properly escape data before storing it in a database, we can make use of the addslashes function. This function serves the purpose of adding escape characters to certain characters that can potentially cause issues when stored. By adding slashes before characters such as single quotes ('), double quotes (") and backslashes (\), we can ensure that the data remains intact and doesn't interfere with the structure or integrity of the database.
Escaping data is essential to prevent any unintended behavior that may arise from special characters being interpreted erroneously. Without proper escaping, certain characters used in SQL queries or other database operations, such as quotes or backslashes, can cause errors or even be exploited for malicious purposes. By adding slashes before these characters, we effectively neutralize their impact and maintain the integrity and security of our stored data.
It's important to note that while addslashes is a common method for escape data in PHP, other programming languages and frameworks may offer different functions or techniques. It is always recommended to consult the documentation specific to the language or framework you are working with to ensure you are using the appropriate method for escaping data in your particular context.
In summary, escaping data before storing it in a database is an important step in maintaining data integrity and security. By utilizing functions like addslashes, we can ensure that special characters are properly handled and stored, minimizing the risk of errors or security vulnerabilities.
What does the unset() function mean?
The unset() function is a dedicated tool in programming for managing variables. When this function is applied to a variable, it effectively renders it undefined, removing any assigned value or data associated with it. In other words, unset() essentially erases the existence of a variable within the program. This can be useful for freeing up memory or when there is a need to remove a variable from further processing or usage in the code.
What does the unlink() function mean?
The unlink() function is a specific function used in file system management. Its main purpose is to delete or remove a file that is specified as the input. By calling the unlink() function with the filename as an argument, the file will be permanently deleted from the file system, freeing up any disk space that was occupied by it and rendering the file inaccessible.
How do I check if a given variable is empty?
To check if a given variable is empty, you can use the empty() function. The empty() function in PHP is used to determine whether a variable has been assigned a value or not. It returns true if the variable is empty and false if it has any value.
Here's how you can use the empty() function:
1. Declare your variable that you want to check for emptiness.
```php
$variable = '';
```
2. To check if the variable is empty, use the empty() function and pass the variable as an argument.
```php
if (empty($variable)) {
echo "The variable is empty.";
} else {
echo "The variable is not empty.";
}
```
In the example above, if the variable contains an empty string (''), null, 0, false, or an empty array, the empty() function will evaluate to true and the first echo statement will execute. If the variable has any other value, the empty() function will evaluate to false and the second echo statement will execute.
Note that the empty() function does not generate a warning or error if the variable being checked does not exist. It is often used to avoid undefined variable errors.
Remember to use the empty() function whenever you need to check whether a variable has a value or not.
How can we check if the value of a given variable is alphanumeric?
To determine if a given variable holds an alphanumeric value, there is a useful function called ctype_alnum. By utilizing this dedicated function, we can effortlessly check whether the value stored in the variable is alphanumeric or not.
How can we check the value of a given variable is a number?
To check if a given variable is a number, we can utilize the is_numeric() function. This function is specifically designed to determine whether a value is numeric or not. By passing the variable as an argument to the is_numeric() function, it will return a boolean value indicating whether the variable is a number or not. If the variable is indeed a number, the function will return true; otherwise, it will return false. This method is particularly useful when dealing with user input or when verifying the data type of a variable to ensure it meets specific requirements.
How can we access the data sent through the URL with the POST method?
To access the data sent through the URL with the POST method, we can utilize the $_POST array in PHP. After creating a form containing input fields, such as 'var', when the user clicks the submit button, the form data is sent via the POST method. In PHP, we can access this data by using the $_POST superglobal array.
To retrieve the value of a specific input field, such as 'var', we can access it using $_POST['var']. This will give us the value that was submitted in the corresponding form field. By referencing the name attribute of the input field in the square brackets, we can retrieve the desired data.
Using this technique, we can effectively access and utilize the data sent through the URL using the POST method for various purposes in our PHP applications.
How can we access the data sent through the URL with the GET method?
To access the data sent through the URL using the GET method, we can utilize the superglobal array in PHP called $_GET. When making a request, parameters can be included in the URL using the query string format: www.url.com?param1=value1¶m2=value2.
To retrieve these values, we can access the $_GET array, using the name of the parameter as the key. For example, if we have a parameter named "var" with the value "value", we can access it like this:
$variable = $_GET['var'];
After executing this line of code, the variable $variable will contain the value "value" that was passed through the URL. It is important to note that the keys in the $_GET array must match the parameter names exactly.
By utilizing the $_GET array, we can easily access and use the data that is sent through the URL using the GET method in PHP.
How is it possible to know the number of rows returned in the result set?
To determine the number of rows returned in a result set, you can use the function mysqli_num_rows(). This PHP function calculates and returns the count of rows in the specified result set. By invoking this function, you can effectively obtain the total number of rows that exist within the result set. This can be useful in scenarios where you need to perform further actions or make decisions based on the number of rows retrieved from the database query.
How can we connect to a MySQL database from a PHP script?
To connect to a MySQL database from a PHP script, we can use the `mysqli_connect()` function. This function requires the following parameters: the host name, user name, and password for the database.
Here is an example of how to connect to a MySQL database:
```php
$database = mysqli_connect('HOST', 'USER_NAME', 'PASSWORD');
?>
```
After establishing a connection, we need to select the specific database we want to work with using the `mysqli_select_db()` function. This function takes two parameters: the database connection and the name of the database.
Here is an example of how to select a specific database:
```php
mysqli_select_db($database, 'DATABASE_NAME');
?>
```
By executing these steps, we can successfully connect to a MySQL database from a PHP script.
What does the PHP error
The PHP error "Parse error in PHP - unexpected T_variable at line x" indicates a syntax error in the PHP code. This error specifically occurs when the PHP parser encounters an unexpected variable (T_variable) at a particular line (line x) in the code. The parser is unable to continue interpreting and executing the program beyond this point, resulting in the error message being displayed. It is essential to carefully review the code at the specified line to identify and correct the mistake, such as missing semicolons, incorrect variable declarations, or mismatched parentheses. Resolving the syntax error will allow the PHP code to be parsed and executed correctly.
How can we display information of a variable and readable by a human with PHP?
To display information of a variable in a readable format with PHP, we can make use of the "print_r()" function. This function allows us to output the content of a variable in a human-readable manner. By passing the variable as an argument to the "print_r()" function, PHP will generate and display a result that provides a clear representation of the variable's contents.
How can I display text with a PHP script?
To display text with a PHP script, there are several methods you can use. Here are three commonly used methods:
Method 1: Using echo
The echo statement is a simple way to display text in PHP. You can use it like this:
```php
echo "This is some text.";
?>
```
In the example above, the text "This is some text." will be displayed on the webpage or outputted in the console, depending on where the PHP script is being executed.
Method 2: Using print
Similar to echo, the print statement can also be used to display text in PHP. Here is an example:
```php
print "This is some text.";
?>
```
The output of this code will be the same as the previous example, displaying the text "This is some text.".
Method 3: Using heredoc or nowdoc syntax
Heredoc and nowdoc syntax provide a convenient way to display multiline text. Here's an example using heredoc syntax:
```php
$text = <<<EOT
This is some text.
It can span multiple lines.
EOT;
echo $text;
?>
```
In this example, the text enclosed between `<<<EOT` and `EOT;` will be displayed as output. You can also use nowdoc syntax, which is similar but doesn't interpret variables or special characters within the text.
These methods allow you to display text in a PHP script. You can choose the one that suits your needs based on simplicity or specific formatting requirements.
What are the functions to be used to get the image's properties (size, width, and height)?
To obtain the image's properties such as its size, width, and height, the following functions need to be used:
1. getimagesize(): This function is used to retrieve the size of the image and provides information like width, height, and type of the image.
2. imagesx(): This function specifically returns the width of the image. It allows you to easily retrieve the width value without any additional information.
3. imagesy(): On the other hand, this function returns the height of the image. Similar to imagesx(), imagesy() exclusively provides the height value of the image.
What is needed to be able to use image functions?
To utilize image functions, one must have the GD library installed on their system. The GD library plays a crucial role in executing these image functions effectively.
What type of operation is needed when passing values through a form or an URL?
When passing values through a form or an URL, it is necessary to perform encoding and decoding operations. These operations ensure that the values are accurately transmitted and can be properly interpreted at the receiving end. To encode values, the htmlspecialchars() function is used, which represents special characters in a way that they won't conflict with HTML or XML syntax. On the other hand, the urlencode() function is used to encode values for URLs, replacing any special characters with their appropriate encoding. Decoding is the reverse process, where the htmlspecialchars_decode() function is employed to convert encoded special characters back to their original form, and the urldecode() function is used to revert URL-encoded values to their initial state. Both encoding and decoding are essential when passing values through a form or an URL to ensure data integrity and proper interpretation.
How to run the interactive PHP shell from the command line interface?
To run the interactive PHP shell from the command line interface, you can follow the steps below:
1. Open your command line interface (such as Terminal on macOS or Command Prompt on Windows).
2. Ensure that PHP is installed on your system. You can check this by running the command `php -v` in the command line. If PHP is installed, you will see the version number. If not, you will need to install PHP before proceeding.
3. Once you have confirmed PHP is installed, use the PHP CLI program to launch the interactive shell. You can do this by running the command `php -a` in the command line.
4. After entering the command, press Enter to execute it. This will start the interactive PHP shell.
5. You can now start entering PHP code directly into the interactive shell and execute it by pressing Enter. Each line you enter will be immediately interpreted and executed by the PHP interpreter.
6. To exit the interactive shell, type `exit` and press Enter.
By following these steps, you will be able to run the interactive PHP shell from the command line interface.
What is the actually used PHP version?
The currently used PHP version is either 7.1 or 7.2, as they are the recommended versions.
Which programming language does PHP resemble?
Which programming language does PHP resemble?
Upcoming PHP Training Certification Online classes
Batch starts on 21st Jun 2025 |
|
||
Batch starts on 25th Jun 2025 |
|
||
Batch starts on 29th Jun 2025 |
|